Advisory boards aren’t only for executives. Join the LogRocket Content Advisory Board today →
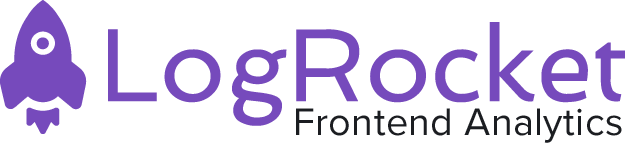
- Product Management
- Solve User-Reported Issues
- Find Issues Faster
- Optimize Conversion and Adoption
- Start Monitoring for Free
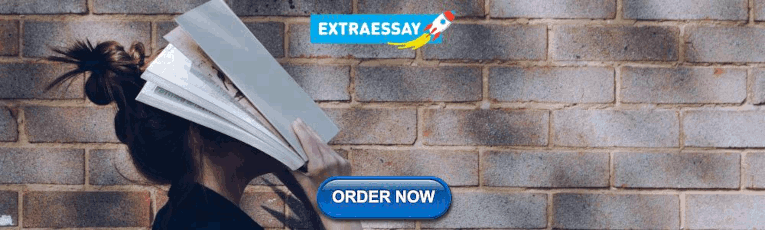
How to dynamically assign properties to an object in TypeScript
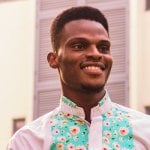
Editor’s note: This article was updated on 6 October 2023, introducing solutions like type assertions and the Partial utility type to address the TypeScript error highlighted.
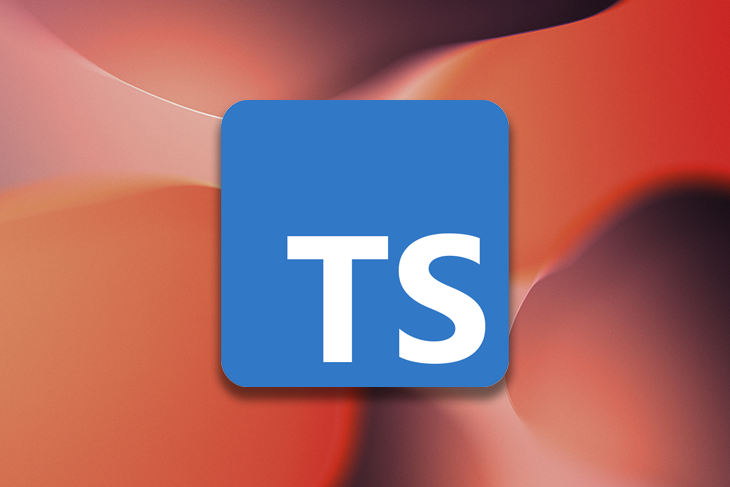
JavaScript is a dynamically typed language, meaning that a variable’s type is determined at runtime and by what it holds at the time of execution. This makes it flexible but also unreliable and prone to errors because a variable’s value might be unexpected.
TypeScript, on the other hand, is a statically typed version of JavaScript — unlike JavaScript, where a variable can change types randomly, TypeScript defines the type of a variable at its declaration or initialization.
Dynamic property assignment is the ability to add properties to an object only when they are needed. This can occur when an object has certain properties set in different parts of our code that are often conditional.
In this article, we will explore some ways to enjoy the dynamic benefits of JavaScript alongside the security of TypeScript’s typing in dynamic property assignment.
Consider the following example of TypeScript code:
This seemingly harmless piece of code throws a TypeScript error when dynamically assigning name to the organization object:
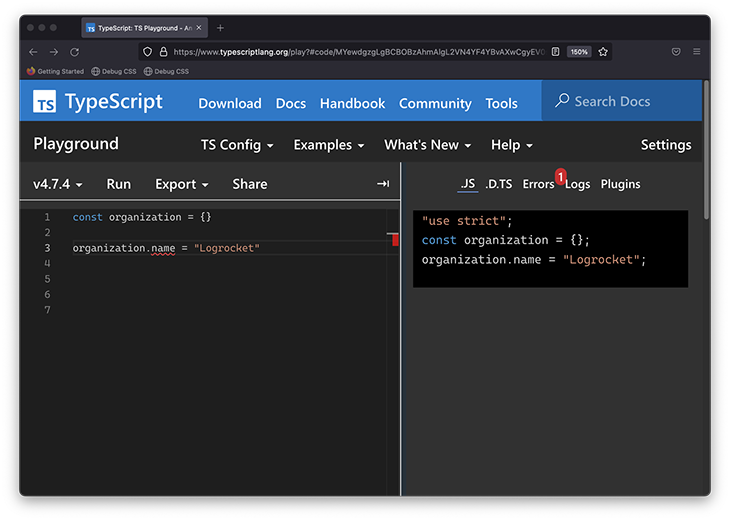
See this example in the TypeScript Playground .
The source of confusion, perhaps rightly justified if you’re a TypeScript beginner, is: how could something that seems so simple be such a problem in TypeScript?
The TL;DR of it all is that if you can’t define the variable type at declaration time, you can use the Record utility type or an object index signature to solve this. But in this article, we’ll go through the problem itself and work toward a solution that should work in most cases.
The problem with dynamically assigning properties to objects
Generally speaking, TypeScript determines the type of a variable when it is declared. This determined type stays the same throughout your application. There are exceptions to this rule, such as when considering type narrowing or working with the any type, but otherwise, this is a general rule to remember.
In the earlier example, the organization object is declared as follows:
There is no explicit type assigned to this variable, so TypeScript infers a type of organization based on the declaration to be {} , i.e., the literal empty object.
If you add a type alias, you can explore the type of organization :
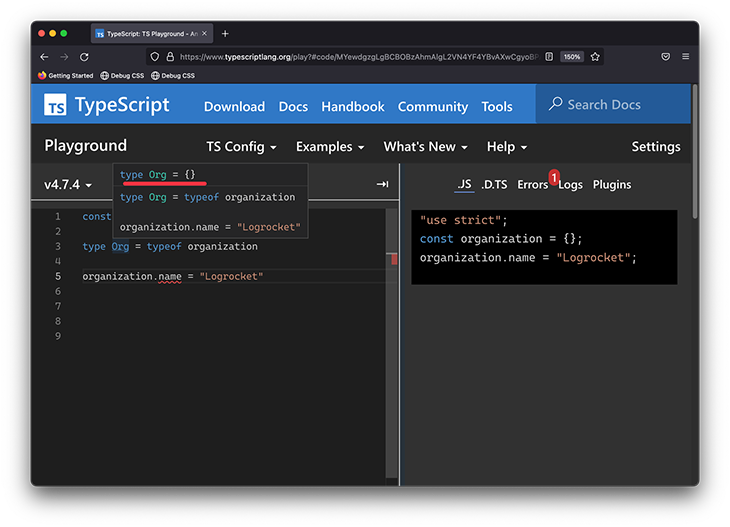
See this in the TypeScript Playground .
When you then try to reference the name prop on this empty object literal:
You receive the following error:
There are many ways to solve the TypeScript error here. Let’s consider the following:
Solution 1: Explicitly type the object at declaration time
This is the easiest solution to reason through. At the time you declare the object, go ahead and type it, and assign all the relevant values:
This eliminates any surprises. You’re clearly stating what this object type is and rightly declaring all relevant properties when you create the object.
However, this is not always feasible if the object properties must be added dynamically, which is why we’re here.
Solution 2: Use an object index signature
Occasionally, the properties of the object truly need to be added at a time after they’ve been declared. In this case, you can use the object index signature, as follows:
When the organization variable is declared, you can explicitly type it to the following: {[key: string] : string} .
You might be used to object types having fixed property types:
However, you can also substitute name for a “variable type.” For example, if you want to define any string property on obj :
Note that the syntax is similar to how you’d use a variable object property in standard JavaScript:
The TypeScript equivalent is called an object index signature.
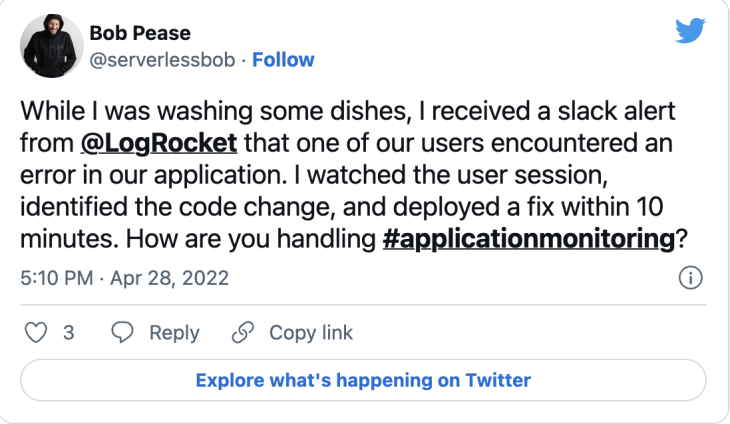
Over 200k developers use LogRocket to create better digital experiences

Moreover, note that you could type key with other primitives:
Solution 3: Use the Record Utility Type
The Record utility type allows you to constrict an object type whose properties are Keys and property values are Type . It has the following signature: Record<Keys, Type> .
In our example, Keys represents string and Type . The solution here is shown below:
Instead of using a type alias, you can also inline the type:
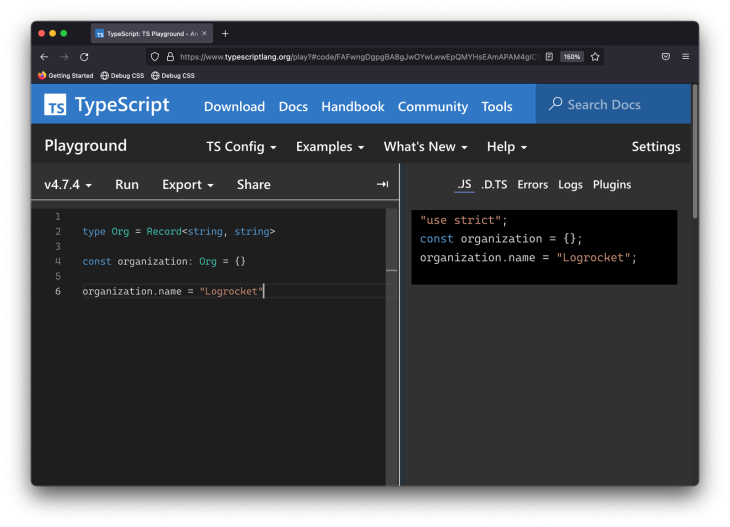
Solution 4: Use the Map data type
A Map object is a fundamentally different data structure from an object , but for completeness, you could eliminate this problem if you were using Map .
Consider the starting example rewritten to use a Map object:
With Map objects, you’ll have no errors when dynamically assigning properties to the object:
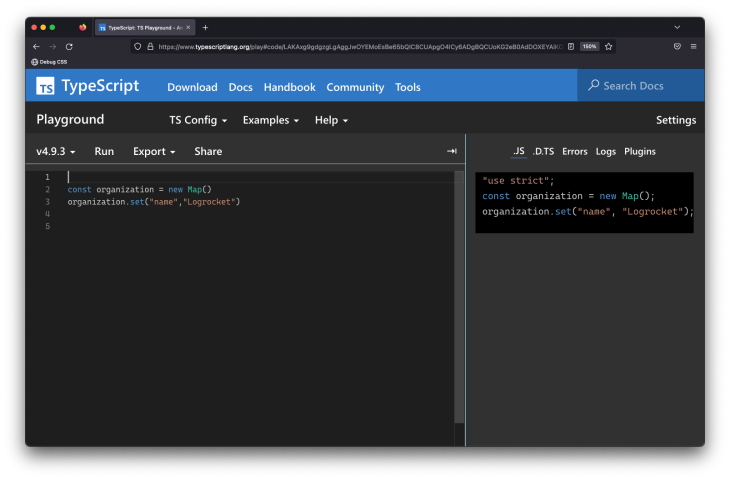
This seems like a great solution at first, but the caveat is your Map object is weakly typed. You can access a nonexisting property and get no warnings at all:
See the TypeScript Playground .
This is unlike the standard object. By default, the initialized Map has the key and value types as any — i.e., new () => Map<any, any> . Consequently, the return type of the s variable will be any :
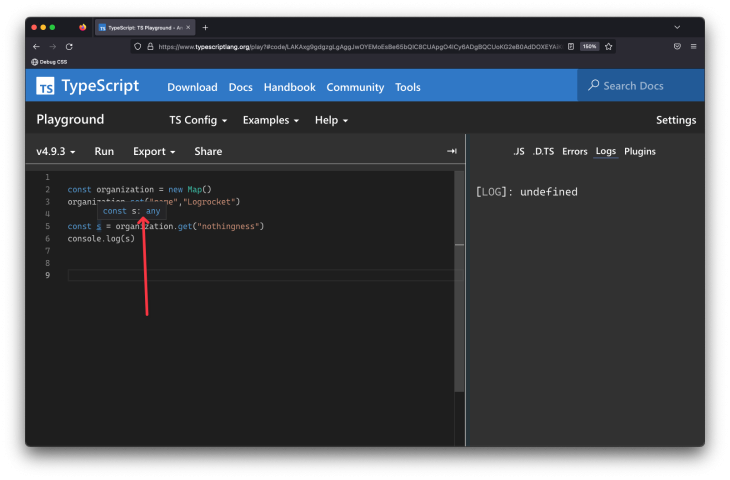
When using Map , at the very least, I strongly suggest passing some type information upon creation. For example:
s will still be undefined, but you won’t be surprised by its code usage. You’ll now receive the appropriate type for it:
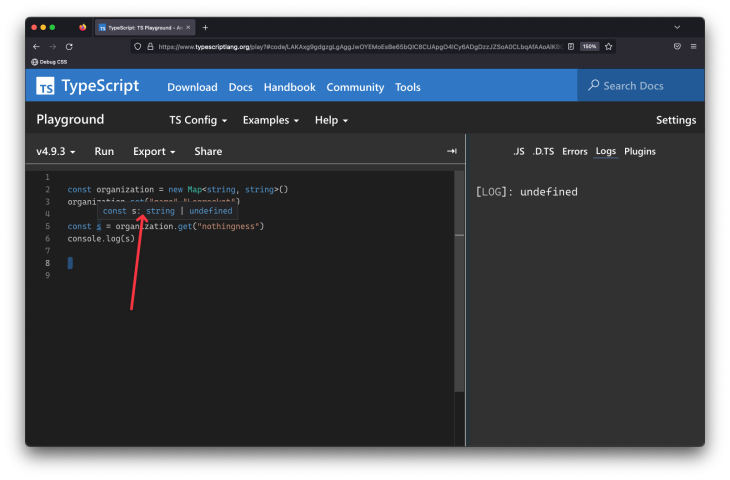
If you truly don’t know what the keys of the Map will be, you can go ahead and represent this at the type level:
And if you’re not sure what the keys or values are, be safe by representing this at the type level:
Solution 5: Consider an optional object property
This solution won’t always be possible, but if you know the name of the property to be dynamically assigned, you can optionally provide this when initializing the object as shown below:
If you don’t like the idea of using optional properties, you can be more explicit with your typing as shown below:
Solution 6: Leveraging type assertions
TypeScript type assertion is a mechanism that tells the compiler the variable’s type and overrides what it infers from the declaration or assignment. With this, we are telling the compiler to trust our understanding of the type because there will be no type verification.
We can perform a type assertion by either using the <> brackets or the as keyword. This is particularly helpful with the dynamic property assignment because it allows the properties we want for our object to be dynamically set because TypeScript won’t enforce them.
Let’s take a look at applying type assertions to our problem case:
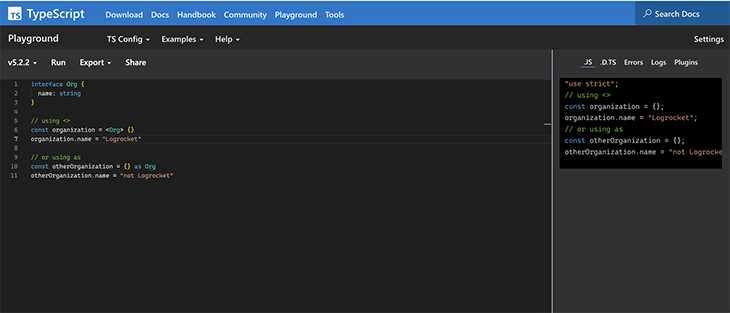
Note that with type assertions, the compiler is trusting that we will enforce the type we have asserted. This means if we don’t, for example, set a value for organization.name , it will throw an error at runtime that we will have to handle ourselves.
Solution 7: Use the Partial utility type
TypeScript provides several utility types that can be used to manipulate types. Some of these utility types are Partial , Omit , Required , and Pick .
For dynamic property assignments, we will focus specifically on the Partial utility type. This takes a defined type and makes all its properties optional. Thus, we can initialize our object with any combination of its properties, from none to all, as each one is optional:
In our example with the Partial utility type, we defined our organization object as the type partial Org , which means we can choose not to set a phoneNumber property:
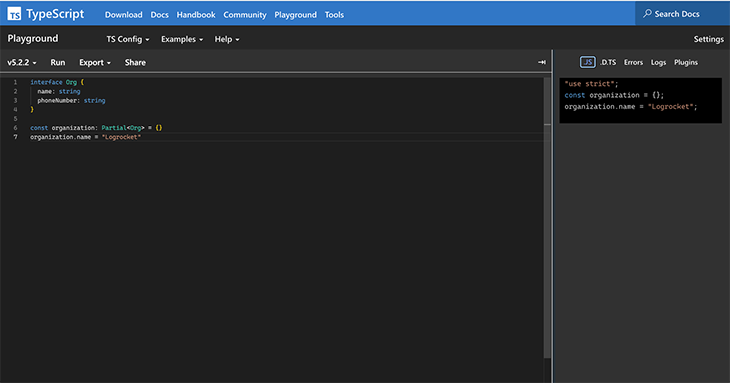
Grouping and comparing the options for adding properties in TypeScript
In this article, we explored the different options for setting properties dynamically in TypeScript. These options can be grouped together by their similarities.
Index/Key signatures
This group of options allows you to define the type of keys allowed without limiting what possible keys can exist. The options in this group include:
- Using an object index signature
- Using the Record utility type
- Using the Map data type (with key/value typing)
With these, we can define that our object will take string indexes and decide what types to support as values, like String , Number , Boolean , or Any :
See in TypeScript Playground .
Pro: The main benefit of these methods is the ability to dynamically add properties to an object while still setting expectations for the potential types of keys and values.
Con: The main disadvantage of this way of defining objects is that you can’t predict what keys our objects will have and so some references may or may not be defined. An additional disadvantage is that if we decide to define our key signature with type Any , then the object becomes even more unpredictable.
Conditional/Optional properties
This set of object assignment methods shares a common feature: the definition of optional properties. This means that the range of possible properties are known but some may or may not be set. The options in this group include:
- Using optional object properties
- Using the Partial utility type
- Using type assertions
See this example in the TypeScript Playground , or in the code block below:
Note: While these options mean that the possible keys are known and may not be set, TypeScript’s compiler won’t validate undefined states when using type assertions. This can lead to unhandled exceptions during runtime. For example, with optional properties and the Partial utility type, name has type string or undefined . Meanwhile, with type assertions, name has type string .
Pro: The advantage of this group of options is that all possible object keys and values are known.
Con: The disadvantage is that while the possible keys are known, we don’t know if those keys have been set and will have to handle the possibility that they are undefined.
Apart from primitives, the most common types you’ll have to deal with are likely object types. In cases where you need to build an object dynamically, take advantage of the Record utility type or use the object index signature to define the allowed properties on the object.
If you’d like to read more on this subject, feel free to check out my cheatsheet on the seven most-asked TypeScript questions on Stack Overflow, or tweet me any questions . Cheers!
LogRocket : Full visibility into your web and mobile apps
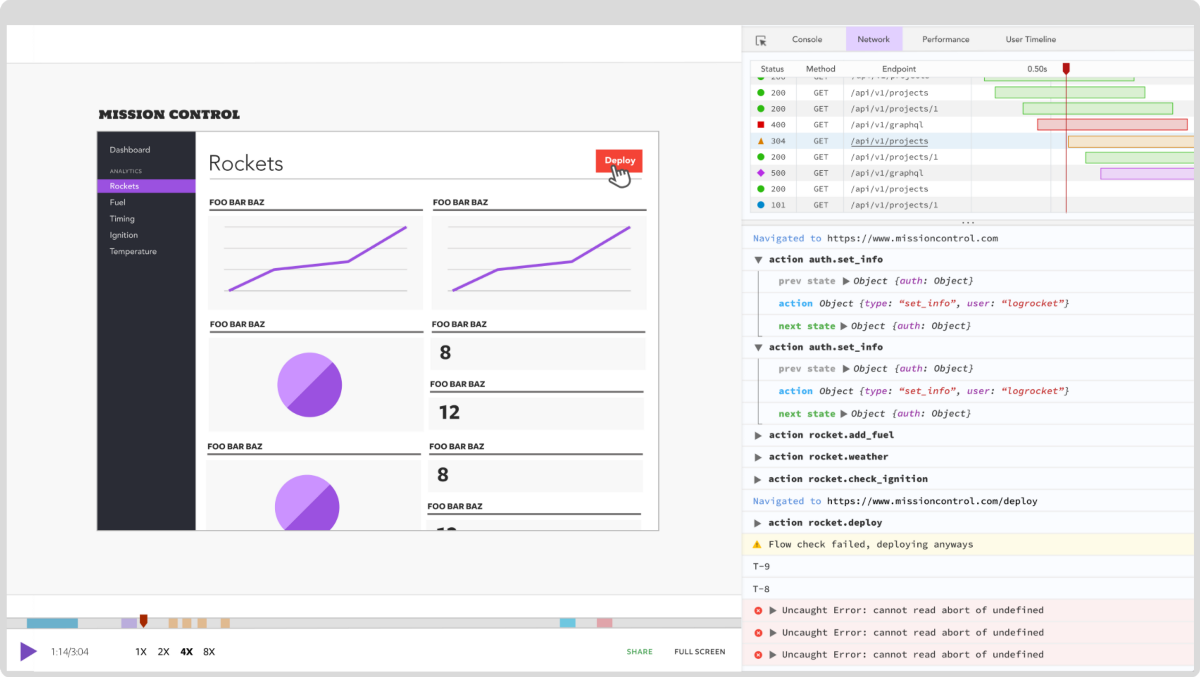
LogRocket is a frontend application monitoring solution that lets you replay problems as if they happened in your own browser. Instead of guessing why errors happen or asking users for screenshots and log dumps, LogRocket lets you replay the session to quickly understand what went wrong. It works perfectly with any app, regardless of framework, and has plugins to log additional context from Redux, Vuex, and @ngrx/store.
In addition to logging Redux actions and state, LogRocket records console logs, JavaScript errors, stacktraces, network requests/responses with headers + bodies, browser metadata, and custom logs. It also instruments the DOM to record the HTML and CSS on the page, recreating pixel-perfect videos of even the most complex single-page and mobile apps.
Try it for free .
Share this:
- Click to share on Twitter (Opens in new window)
- Click to share on Reddit (Opens in new window)
- Click to share on LinkedIn (Opens in new window)
- Click to share on Facebook (Opens in new window)
- #typescript
Hey there, want to help make our blog better?
Join LogRocket’s Content Advisory Board. You’ll help inform the type of content we create and get access to exclusive meetups, social accreditation, and swag.
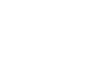
Stop guessing about your digital experience with LogRocket
Recent posts:.
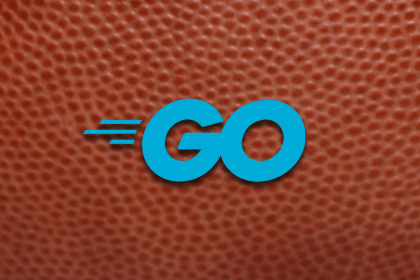
Go long by generating PDFs in Golang with Maroto
Go, also known as Golang, is a statically typed, compiled programming language designed by Google. It combines the performance and […]
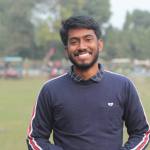
MobX adoption guide: Overview, examples, and alternatives
MobX is a lightweight, boilerplate-free state management library that offers a reactive approach to state management.
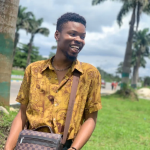
Hydration visualization in Angular 18 using debugging tools
Angular 18’s hydration error visualization boosts application debugging by clearly identifying which components are fully hydrated.
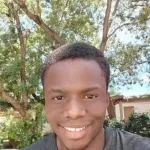
Turbopack adoption guide: Overview, examples, and alternatives
Turbopack is a next-generation incremental bundler optimized for JavaScript and TypeScript. Let’s explore why and how to adopt it.
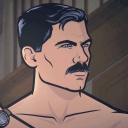
3 Replies to "How to dynamically assign properties to an object in TypeScript"
I know this is explicitly for TypeScript, and I think type declarations should always be first. But in general, you can also use a Map object. If it’s really meant to by dynamic, might as well utilize the power of Map.
Great suggestion (updated the article). It’s worth mentioning the weak typing you get by default i.e., with respect to Typescript.
Hi, thanks for your valuable article please consider ‘keyof type’ in TypeScript, and add this useful solution if you are happy have nice time
Leave a Reply Cancel reply
11 Properties: assignment vs. definition
- 11.1.1 Assignment
- 11.1.2 Definition
- 11.2.1 Assigning to a property
- 11.2.2 Defining a property
- 11.3.1 Only definition allows us to create a property with arbitrary attributes
- 11.3.2 The assignment operator does not change properties in prototypes
- 11.3.3 Assignment calls setters, definition doesn’t
- 11.3.4 Inherited read-only properties prevent creating own properties via assignment
- 11.4.1 The properties of an object literal are added via definition
- 11.4.2 The assignment operator = always uses assignment
- 11.4.3 Public class fields are added via definition
- 11.5 Further reading and sources of this chapter
There are two ways of creating or changing a property prop of an object obj :
- Assigning : obj.prop = true
- Defining : Object.defineProperty(obj, '', {value: true})
This chapter explains how they work.
For this chapter, you should be familiar with property attributes and property descriptors. If you aren’t, check out §9 “Property attributes: an introduction” .
11.1 Assignment vs. definition
11.1.1 assignment.
We use the assignment operator = to assign a value value to a property .prop of an object obj :
This operator works differently depending on what .prop looks like:
Changing properties: If there is an own data property .prop , assignment changes its value to value .
Invoking setters: If there is an own or inherited setter for .prop , assignment invokes that setter.
Creating properties: If there is no own data property .prop and no own or inherited setter for it, assignment creates a new own data property.
That is, the main purpose of assignment is making changes. That’s why it supports setters.
11.1.2 Definition
To define a property with the key propKey of an object obj , we use an operation such as the following method:
This method works differently depending on what the property looks like:
- Changing properties: If an own property with key propKey exists, defining changes its property attributes as specified by the property descriptor propDesc (if possible).
- Creating properties: Otherwise, defining creates an own property with the attributes specified by propDesc (if possible).
That is, the main purpose of definition is to create an own property (even if there is an inherited setter, which it ignores) and to change property attributes.
11.2 Assignment and definition in theory (optional)
In specification operations, property descriptors are not JavaScript objects but Records , a spec-internal data structure that has fields . The keys of fields are written in double brackets. For example, Desc.[[Configurable]] accesses the field .[[Configurable]] of Desc . These records are translated to and from JavaScript objects when interacting with the outside world.
11.2.1 Assigning to a property
The actual work of assigning to a property is handled via the following operation in the ECMAScript specification:
These are the parameters:
- O is the object that is currently being visited.
- P is the key of the property that we are assigning to.
- V is the value we are assigning.
- Receiver is the object where the assignment started.
- ownDesc is the descriptor of O[P] or null if that property doesn’t exist.
The return value is a boolean that indicates whether or not the operation succeeded. As explained later in this chapter , strict-mode assignment throws a TypeError if OrdinarySetWithOwnDescriptor() fails.
This is a high-level summary of the algorithm:
- It traverses the prototype chain of Receiver until it finds a property whose key is P . The traversal is done by calling OrdinarySetWithOwnDescriptor() recursively. During recursion, O changes and points to the object that is currently being visited, but Receiver stays the same.
- Depending on what the traversal finds, an own property is created in Receiver (where recursion started) or something else happens.
In more detail, this algorithm works as follows:
If O has a prototype parent , then we return parent.[[Set]](P, V, Receiver) . This continues our search. The method call usually ends up invoking OrdinarySetWithOwnDescriptor() recursively.
Otherwise, our search for P has failed and we set ownDesc as follows:
With this ownDesc , the next if statement will create an own property in Receiver .
- If ownDesc.[[Writable]] is false , return false . This means that any non-writable property P (own or inherited!) prevents assignment.
- The current object O and the current property descriptor ownDesc on one hand.
- The original object Receiver and the original property descriptor existingDescriptor on the other hand.
- (If we get here, then we are still at the beginning of the prototype chain – we only recurse if Receiver does not have a property P .)
- If existingDescriptor specifies an accessor, return false .
- If existingDescriptor.[[Writable]] is false , return false .
- Return Receiver.[[DefineOwnProperty]](P, { [[Value]]: V }) . This internal method performs definition, which we use to change the value of property Receiver[P] . The definition algorithm is described in the next subsection.
- (If we get here, then Receiver does not have an own property with key P .)
- Return CreateDataProperty(Receiver, P, V) . ( This operation creates an own data property in its first argument.)
- (If we get here, then ownDesc describes an accessor property that is own or inherited.)
- Let setter be ownDesc.[[Set]] .
- If setter is undefined , return false .
- Perform Call(setter, Receiver, «V») . Call() invokes the function object setter with this set to Receiver and the single parameter V (French quotes «» are used for lists in the specification).
Return true .
11.2.1.1 How do we get from an assignment to OrdinarySetWithOwnDescriptor() ?
Evaluating an assignment without destructuring involves the following steps:
- In the spec, evaluation starts in the section on the runtime semantics of AssignmentExpression . This section handles providing names for anonymous functions, destructuring, and more.
- If there is no destructuring pattern, then PutValue() is used to make the assignment.
- For property assignments, PutValue() invokes the internal method .[[Set]]() .
- For ordinary objects, .[[Set]]() calls OrdinarySet() (which calls OrdinarySetWithOwnDescriptor() ) and returns the result.
Notably, PutValue() throws a TypeError in strict mode if the result of .[[Set]]() is false .
11.2.2 Defining a property
The actual work of defining a property is handled via the following operation in the ECMAScript specification:
The parameters are:
- The object O where we want to define a property. There is a special validation-only mode where O is undefined . We are ignoring this mode here.
- The property key P of the property we want to define.
- extensible indicates if O is extensible.
- Desc is a property descriptor specifying the attributes we want the property to have.
- current contains the property descriptor of an own property O[P] if it exists. Otherwise, current is undefined .
The result of the operation is a boolean that indicates if it succeeded. Failure can have different consequences. Some callers ignore the result. Others, such as Object.defineProperty() , throw an exception if the result is false .
This is a summary of the algorithm:
If current is undefined , then property P does not currently exist and must be created.
- If extensible is false , return false indicating that the property could not be added.
- Otherwise, check Desc and create either a data property or an accessor property.
If Desc doesn’t have any fields, return true indicating that the operation succeeded (because no changes had to be made).
If current.[[Configurable]] is false :
- ( Desc is not allowed to change attributes other than value .)
- If Desc.[[Configurable]] exists, it must have the same value as current.[[Configurable]] . If not, return false .
- Same check: Desc.[[Enumerable]]
Next, we validate the property descriptor Desc : Can the attributes described by current be changed to the values specified by Desc ? If not, return false . If yes, go on.
- If the descriptor is generic (with no attributes specific to data properties or accessor properties), then validation is successful and we can move on.
- The current property must be configurable (otherwise its attributes can’t be changed as necessary). If not, false is returned.
- Change the current property from a data property to an accessor property or vice versa. When doing so, the values of .[[Configurable]] and .[[Enumerable]] are preserved, all other attributes get default values ( undefined for object-valued attributes, false for boolean-valued attributes).
- (Due to current.[[Configurable]] being false , Desc.[[Configurable]] and Desc.[[Enumerable]] were already checked previously and have the correct values.)
- If Desc.[[Writable]] exists and is true , then return false .
- If Desc.[[Value]] exists and does not have the same value as current.[[Value]] , then return false .
- There is nothing more to do. Return true indicating that the algorithm succeeded.
- (Note that normally, we can’t change any attributes of a non-configurable property other than its value. The one exception to this rule is that we can always go from writable to non-writable. This algorithm handles this exception correctly.)
- If Desc.[[Set]] exists, it must have the same value as current.[[Set]] . If not, return false .
- Same check: Desc.[[Get]]
Set the attributes of the property with key P to the values specified by Desc . Due to validation, we can be sure that all of the changes are allowed.
11.3 Definition and assignment in practice
This section describes some consequences of how property definition and assignment work.
11.3.1 Only definition allows us to create a property with arbitrary attributes
If we create an own property via assignment, it always creates properties whose attributes writable , enumerable , and configurable are all true .
Therefore, if we want to specify arbitrary attributes, we must use definition.
And while we can create getters and setters inside object literals, we can’t add them later via assignment. Here, too, we need definition.
11.3.2 The assignment operator does not change properties in prototypes
Let us consider the following setup, where obj inherits the property prop from proto .
We can’t (destructively) change proto.prop by assigning to obj.prop . Doing so creates a new own property:
The rationale for this behavior is as follows: Prototypes can have properties whose values are shared by all of their descendants. If we want to change such a property in only one descendant, we must do so non-destructively, via overriding. Then the change does not affect the other descendants.
11.3.3 Assignment calls setters, definition doesn’t
What is the difference between defining the property .prop of obj versus assigning to it?
If we define, then our intention is to either create or change an own (non-inherited) property of obj . Therefore, definition ignores the inherited setter for .prop in the following example:
If, instead, we assign to .prop , then our intention is often to change something that already exists and that change should be handled by the setter:
11.3.4 Inherited read-only properties prevent creating own properties via assignment
What happens if .prop is read-only in a prototype?
In any object that inherits the read-only .prop from proto , we can’t use assignment to create an own property with the same key – for example:
Why can’t we assign? The rationale is that overriding an inherited property by creating an own property can be seen as non-destructively changing the inherited property. Arguably, if a property is non-writable, we shouldn’t be able to do that.
However, defining .prop still works and lets us override:
Accessor properties that don’t have a setter are also considered to be read-only:
The fact that read-only properties prevent assignment earlier in the prototype chain, has been given the name override mistake :
- It was introduced in ECMAScript 5.1.
- On one hand, this behavior is consistent with how prototypal inheritance and setters work. (So, arguably, it is not a mistake.)
- On the other hand, with the behavior, deep-freezing the global object causes unwanted side-effects.
- There was an attempt to change the behavior, but that broke the library Lodash and was abandoned ( pull request on GitHub ).
- Pull request on GitHub
- Wiki page on ECMAScript.org ( archived )
11.4 Which language constructs use definition, which assignment?
In this section, we examine where the language uses definition and where it uses assignment. We detect which operation is used by tracking whether or not inherited setters are called. See §11.3.3 “Assignment calls setters, definition doesn’t” for more information.
11.4.1 The properties of an object literal are added via definition
When we create properties via an object literal, JavaScript always uses definition (and therefore never calls inherited setters):
11.4.2 The assignment operator = always uses assignment
The assignment operator = always uses assignment to create or change properties.
11.4.3 Public class fields are added via definition
Alas, even though public class fields have the same syntax as assignment, they do not use assignment to create properties, they use definition (like properties in object literals):
11.5 Further reading and sources of this chapter
Section “Prototype chains” in “JavaScript for impatient programmers”
Email by Allen Wirfs-Brock to the es-discuss mailing list : “The distinction between assignment and definition […] was not very important when all ES had was data properties and there was no way for ES code to manipulate property attributes.” [That changed with ECMAScript 5.]
JS Reference
Html events, html objects, other references, javascript object.assign(), description.
The Object.assign() method copies properties from one or more source objects to a target object.
Related Methods:
Object.assign() copies properties from a source object to a target object.
Object.create() creates an object from an existing object.
Object.fromEntries() creates an object from a list of keys/values.
Parameter | Description |
Required. An existing object. | |
Required. One or more sources. |
Return Value
Type | Description |
Object | The target object. |
Advertisement
Browser Support
Object.assign() is an ECMAScript6 (ES6) feature.
ES6 (JavaScript 2015) is supported in all modern browsers since June 2017:
Chrome 51 | Edge 15 | Firefox 54 | Safari 10 | Opera 38 |
May 2016 | Apr 2017 | Jun 2017 | Sep 2016 | Jun 2016 |
Object.assign() is not supported in Internet Explorer.
Object Tutorials
JavaScript Objects
JavaScript Object Definition
JavaScript Object Methods
JavaScript Object Properties

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.

Member-only story
Master object manipulation using object.assign, object.freeze, and proxies: Advanced Javascript

Vamsi Krishna Kodimela
Angular Simplified
O bject manipulation is one of the key concepts used by almost every JavaScript developer. While basic techniques like property assignment and object creation are essential, JavaScript offers more advanced methods for working with objects, providing flexibility, control, and immutability.
In this story, we’ll explore three powerful tools: Object.assign, Object.freeze, and proxies.
Object.assign: The Master of Merging
Object.assign is a built-in method that allows you to merge properties from multiple objects into a target object. It’s highly versatile and serves various purposes:
- Combining objects: Create new objects with properties from multiple sources.
- Cloning objects: Create shallow copies of objects, retaining their structure and values.
- Default values: Set default properties for objects, ensuring they always have certain values.
Key Points:
- Overwrites existing properties in the target object with those from the source objects.
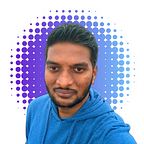
Written by Vamsi Krishna Kodimela
Text to speech
Properties in JavaScript: definition versus assignment
Definition versus assignment.
Assignment. To assign to a property, one uses an expression such as
The following two sections go into more detail regarding how definition and assignment work. Feel free to skip them. You should still be able to understand Sect. 4, “The consequences”, and later.
Recap: property attributes and internal properties
Kinds of properties.
- Named accessor properties: A property that exists thanks to a getter or a setter.
- Named data properties: A property that h as a value. Those are the most common properties. They include methods.
- Internal properties: are used internally by JavaScript and not directly accessible via the language. However, there can be indirect ways of accessing them. Example: Every object has an internal property called [[Prototype]]. You cannot directly read it, but still retrieve its value, via Object.getPrototypeOf() . While internal properties are referred to by a name in square brackets, they are nameless in the sense that they are invisible and don’t have a normal, string-valued property name.
Property attributes
- [[Enumerable]]: If a property is non-enumerable, it can’t be seen by some operations, such as for...in and Object.keys() [2] .
- [[Configurable]]: If a property is non-configurable, none of the attributes (except [[Value]]) can be changed via a definition.
- [[Value]]: is the value of the property.
- [[Writable]]: determines whether the value can be changed.
- [[Get]]: holds a getter method.
- [[Set]]: holds a setter method.
Property descriptors
Internal properties
- [[Prototype]]: The prototype of the object.
- [[Extensible]]: Is this object extensible , can new properties be added to it?
- [[DefineOwnProperty]]: Define a property. See explanation below.
- [[Put]]: Assign to a property. See explanation below.
The details of definition and assignment
Defining a property.
[[ DefineOwnProperty ]] (P, Desc, Throw)
- If this does not have an own property whose name is P : Create a new property if the object is extensible, reject if it isn’t.
- Otherwise, there already is an own property and the definition changes that property.
- Converting a data property to an accessor property or vice versa
- Changing [[Configurable]] or [[Enumerable]]
- Changing [[Writable]]
- Changing [[Value]] if [[Writable]] is false
- Changing [[Get]] or [[Set]]
- Otherwise, the existing own property is configurable and can be changed as specified.
Two functions for defining a property are Object.defineProperty and Object.defineProperties . For example:
Assigning to a property
[[ Put ]] (P, Value, Throw)
- If there is a read-only property whose name is P somewhere in the prototype chain: reject.
- If there is a setter whose name is P somewhere in the prototype chain: call the setter.
- If there is no own property whose name is P : if the the object is extensible then create a new property. this.[[DefineOwnProperty]]( P, { value: Value, writable: true, enumerable: true, configurable: true }, Throw ) If the object is not extensible then reject.
- Otherwise, there is an own property named P that is writable. Invoke this.[[DefineOwnProperty]](P, { value: Value }, Throw) That updates the value of P , but keeps its attributes (such as enumerability) unchanged
The consequences
Assignment calls a setter in a prototype, definition creates an own property, read-only properties in prototypes prevent assignment, but not definition.
Definition. With definition, we want to create a new own property:
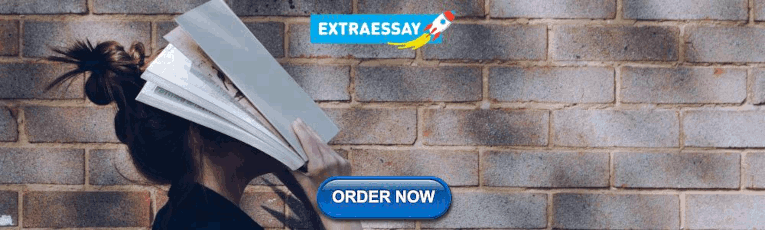
The assignment operator does not change properties in prototypes
- Methods: Allow methods to be patched, directly in the prototype, but prevent accidental changes via descendants of the prototype.
- Non-method properties: The prototype provides shared default values for descendants. One can override these values via a descendant, but not change them. This is considered an anti-pattern and discouraged. It is cleaner to assign default values in constructors.
Only definition allows you to create a property with arbitrary attributes
The properties of an object literal are added via definition, attributes of methods.
- If you want to create a new property, use definition.
- If you want to change the value of a property, use assignment.
- Prototypes as classes – an introduction to JavaScript inheritance
- JavaScript properties: inheritance and enumerability
- Fixing the Read-only Override Prohibition Mistake [a page on the ECMAScript wiki with background information on this issue]
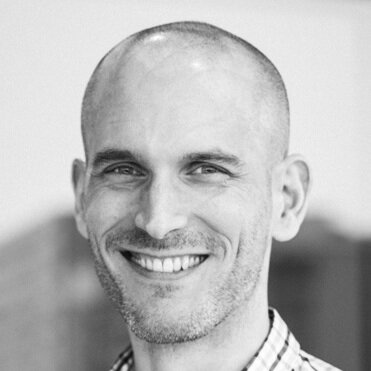

DEV Community

Posted on May 8, 2020 • Updated on May 14, 2020
JS Fundamentals: Object Assignment vs. Primitive Assignment
Introduction.
Something I wish I had understood early on in my JavaScript programming career is how object assignment works and how it's different from primitive assignment. This is my attempt to convey the distinction in the most concise way possible!
Learn JS Fundamentals
Looking to learn more JS fundamentals? Consider signing up for my free mailing list !
Primitives vs. Objects
As a review, let's recall the different primitive types and objects in JavaScript.
Primitive types: Boolean, Null, Undefined, Number, BigInt (you probably won't see this much), String, Symbol (you probably won't see this much)
Object types: Object, Array, Date, Many others
How Primitive and Object Assignment Differ
Primitive assignment.
Assigning a primitive value to a variable is fairly staightforward: the value is assigned to the variable. Let's look at an example.
In this case, a is set to the value hello and b is also set to the value hello . This means if we set b to a new value, a will remain unchanged; there is no relationship between a and b .
Object Assignment
Object assignment works differently. Assigning an object to a variable does the following:
- Creates the object in memory
- Assigns a reference to the object in memory to the variable
Why is this a big deal? Let's explore.
The first line creates the object { name: 'Joe' } in memory and then assigns a reference to that object to variable a . The second line assigns a reference to that same object in memory to b !
So to answer the "why is this a big deal" question, let's mutate a property of the object assigned to b :
That's right! Since a and b are assigned a reference to the same object in memory, mutating a property on b is really just mutating a property on the object in memory that both a and b are pointing to.
To be thorough, we can see this in action with arrays as well.
This Applies to Function Arguments too!
These assignment rules apply when you pass objects to functions too! Check out the following example:
The moral of the story: beware of mutating objects you pass to functions unless this is intended (I don't think there are many instances you'd really want to do this).
Preventing Unintended Mutation
In a lot of cases, this behavior can be desired. Pointing to the same object in memory helps us pass references around and do clever things. However, this is not always the desired behavior, and when you start mutating objects unintentionally you can end up with some very confusing bugs.
There are a few ways to make sure your objects are unique. I'll go over some of them here, but rest assured this list will not be comprehensive.
The Spread Operator (...)
The spread operator is a great way to make a shallow copy of an object or array. Let's use it to copy an object.
A note on "shallow" copying
It's important to understand shallow copying versus deep copying. Shallow copying works well for object that are only one level deep, but nested object become problematic. Let's use the following example:
We successfully copied a one level deep, but the properties at the second level are still referencing the same objects in memory! For this reason, people have invented ways to do "deep" copying, such as using a library like deep-copy or serializing and de-serializing an object.
Using Object.assign
Object.assign can be used to create a new object based on another object. The syntax goes like this:
Beware; this is still a shallow copy!
Serialize and De-serialize
One method that can be used to deep copy an object is to serialize and de-serialize the object. One common way to do this is using JSON.stringify and JSON.parse .
This does have its downsides though. Serializing an de-serializing doesn't preserve complex objects like functions.
A Deep Copy Library
It's fairly common to bring in a deep copy library to do the heavy lifting on this task, especially if your object has an unknown or particularly deep hierarchy. These libraries are typically functions that perform one of the aforementioned shallow copy methods recursively down the object tree.
While this can seem like a complex topic, you'll be just fine if you maintain awareness about how primitive types and objects are assigned differently. Play around with some of these examples and, if you're up for it, attempt writing your own deep copy function!
Top comments (5)
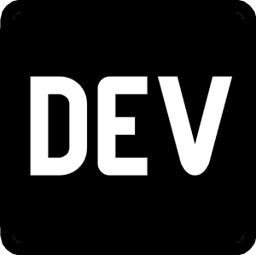
Templates let you quickly answer FAQs or store snippets for re-use.

- Location Online
- Joined Nov 28, 2018
Great article Nick! Well-written, clear, and concise. Really appreciate the images and examples to go along with your main points. This will help a number of devs for sure! Thanks for sharing with us :)

- Location Hamburg, Germany
- Work Senior Frontend Developer at Freelance
- Joined Mar 27, 2020
Great article, thanks alot!!

- Joined Apr 7, 2020
const b = JSON.parse(JSON.serialize(a));
serialize? maybe stringify

- Work DevOps Architect
- Joined Jan 27, 2022

- Location York
- Education Self-taught
- Work Backend Engineer at RotaCloud
- Joined Mar 6, 2018
Nice work 🤩easily explained! Thanks.
Are you sure you want to hide this comment? It will become hidden in your post, but will still be visible via the comment's permalink .
Hide child comments as well
For further actions, you may consider blocking this person and/or reporting abuse
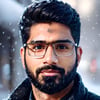
Meet BullMQ
Muneeb Hussain - Aug 7

🙅10 Common React.js Errors and How to Solve Them✨
Pratik Tamhane - Aug 21
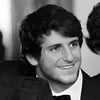
LangChain Part 4 - Leveraging Memory and Storage in LangChain: A Comprehensive Guide
James - Aug 21

Destructuring assignment
The two most used data structures in JavaScript are Object and Array .
- Objects allow us to create a single entity that stores data items by key.
- Arrays allow us to gather data items into an ordered list.
However, when we pass these to a function, we may not need all of it. The function might only require certain elements or properties.
Destructuring assignment is a special syntax that allows us to “unpack” arrays or objects into a bunch of variables, as sometimes that’s more convenient.
Destructuring also works well with complex functions that have a lot of parameters, default values, and so on. Soon we’ll see that.
Array destructuring
Here’s an example of how an array is destructured into variables:
Now we can work with variables instead of array members.
It looks great when combined with split or other array-returning methods:
As you can see, the syntax is simple. There are several peculiar details though. Let’s see more examples to understand it better.
It’s called “destructuring assignment,” because it “destructurizes” by copying items into variables. However, the array itself is not modified.
It’s just a shorter way to write:
Unwanted elements of the array can also be thrown away via an extra comma:
In the code above, the second element of the array is skipped, the third one is assigned to title , and the rest of the array items are also skipped (as there are no variables for them).
…Actually, we can use it with any iterable, not only arrays:
That works, because internally a destructuring assignment works by iterating over the right value. It’s a kind of syntax sugar for calling for..of over the value to the right of = and assigning the values.
We can use any “assignables” on the left side.
For instance, an object property:
In the previous chapter, we saw the Object.entries(obj) method.
We can use it with destructuring to loop over the keys-and-values of an object:
The similar code for a Map is simpler, as it’s iterable:
There’s a well-known trick for swapping values of two variables using a destructuring assignment:
Here we create a temporary array of two variables and immediately destructure it in swapped order.
We can swap more than two variables this way.
The rest ‘…’
Usually, if the array is longer than the list at the left, the “extra” items are omitted.
For example, here only two items are taken, and the rest is just ignored:
If we’d like also to gather all that follows – we can add one more parameter that gets “the rest” using three dots "..." :
The value of rest is the array of the remaining array elements.
We can use any other variable name in place of rest , just make sure it has three dots before it and goes last in the destructuring assignment.
Default values
If the array is shorter than the list of variables on the left, there will be no errors. Absent values are considered undefined:
If we want a “default” value to replace the missing one, we can provide it using = :
Default values can be more complex expressions or even function calls. They are evaluated only if the value is not provided.
For instance, here we use the prompt function for two defaults:
Please note: the prompt will run only for the missing value ( surname ).
Object destructuring
The destructuring assignment also works with objects.
The basic syntax is:
We should have an existing object on the right side, that we want to split into variables. The left side contains an object-like “pattern” for corresponding properties. In the simplest case, that’s a list of variable names in {...} .
For instance:
Properties options.title , options.width and options.height are assigned to the corresponding variables.
The order does not matter. This works too:
The pattern on the left side may be more complex and specify the mapping between properties and variables.
If we want to assign a property to a variable with another name, for instance, make options.width go into the variable named w , then we can set the variable name using a colon:
The colon shows “what : goes where”. In the example above the property width goes to w , property height goes to h , and title is assigned to the same name.
For potentially missing properties we can set default values using "=" , like this:
Just like with arrays or function parameters, default values can be any expressions or even function calls. They will be evaluated if the value is not provided.
In the code below prompt asks for width , but not for title :
We also can combine both the colon and equality:
If we have a complex object with many properties, we can extract only what we need:
The rest pattern “…”
What if the object has more properties than we have variables? Can we take some and then assign the “rest” somewhere?
We can use the rest pattern, just like we did with arrays. It’s not supported by some older browsers (IE, use Babel to polyfill it), but works in modern ones.
It looks like this:
In the examples above variables were declared right in the assignment: let {…} = {…} . Of course, we could use existing variables too, without let . But there’s a catch.
This won’t work:
The problem is that JavaScript treats {...} in the main code flow (not inside another expression) as a code block. Such code blocks can be used to group statements, like this:
So here JavaScript assumes that we have a code block, that’s why there’s an error. We want destructuring instead.
To show JavaScript that it’s not a code block, we can wrap the expression in parentheses (...) :
Nested destructuring
If an object or an array contains other nested objects and arrays, we can use more complex left-side patterns to extract deeper portions.
In the code below options has another object in the property size and an array in the property items . The pattern on the left side of the assignment has the same structure to extract values from them:
All properties of options object except extra which is absent in the left part, are assigned to corresponding variables:
Finally, we have width , height , item1 , item2 and title from the default value.
Note that there are no variables for size and items , as we take their content instead.
Smart function parameters
There are times when a function has many parameters, most of which are optional. That’s especially true for user interfaces. Imagine a function that creates a menu. It may have a width, a height, a title, an item list and so on.
Here’s a bad way to write such a function:
In real-life, the problem is how to remember the order of arguments. Usually, IDEs try to help us, especially if the code is well-documented, but still… Another problem is how to call a function when most parameters are ok by default.
That’s ugly. And becomes unreadable when we deal with more parameters.
Destructuring comes to the rescue!
We can pass parameters as an object, and the function immediately destructurizes them into variables:
We can also use more complex destructuring with nested objects and colon mappings:
The full syntax is the same as for a destructuring assignment:
Then, for an object of parameters, there will be a variable varName for the property incomingProperty , with defaultValue by default.
Please note that such destructuring assumes that showMenu() does have an argument. If we want all values by default, then we should specify an empty object:
We can fix this by making {} the default value for the whole object of parameters:
In the code above, the whole arguments object is {} by default, so there’s always something to destructurize.
Destructuring assignment allows for instantly mapping an object or array onto many variables.
The full object syntax:
This means that property prop should go into the variable varName and, if no such property exists, then the default value should be used.
Object properties that have no mapping are copied to the rest object.
The full array syntax:
The first item goes to item1 ; the second goes into item2 , and all the rest makes the array rest .
It’s possible to extract data from nested arrays/objects, for that the left side must have the same structure as the right one.
We have an object:
Write the destructuring assignment that reads:
- name property into the variable name .
- years property into the variable age .
- isAdmin property into the variable isAdmin (false, if no such property)
Here’s an example of the values after your assignment:
The maximal salary
There is a salaries object:
Create the function topSalary(salaries) that returns the name of the top-paid person.
- If salaries is empty, it should return null .
- If there are multiple top-paid persons, return any of them.
P.S. Use Object.entries and destructuring to iterate over key/value pairs.
Open a sandbox with tests.
Open the solution with tests in a sandbox.
Lesson navigation
- © 2007—2024 Ilya Kantor
- about the project
- terms of usage
- privacy policy
- Environment
- National Politics
- Investigations
- Florida Voices
Real estate firm objects to proposed $439M acquisition of Steward Health Care
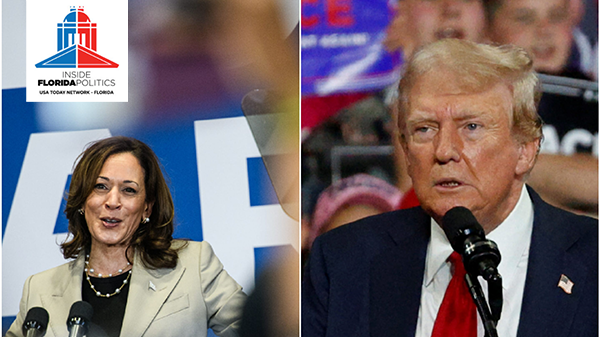
TALLAHASSEE — A real-estate firm has objected to a proposed $439 million deal that would lead to the health system Orlando Health buying three hospitals in Brevard and Indian River counties as part of the bankruptcy of hospital operator Steward Health Care.
Medical Properties Trust, Inc., which owns the hospital properties and leases them to Steward, argued in a court document Monday that Steward had not properly followed bidding procedures because it did not distinguish between the values of hospital operations and the real estate.
The document said bankruptcy laws and the U.S. Constitution don’t allow Steward and Orlando Health to “dictate the terms on which the debtor’s landlord (MPT) will sell its private property.”
“There is no basis at all for a scheme under which a non-debtor real estate owner can be compelled not just to sell its property, but to accept whatever (capped) price that the buyer dictates,” MPT’s attorneys wrote.
But Steward’s attorneys Monday filed a complaint that said Steward and its affiliates, “consistent with their rights under the bidding procedures, allowed bidders to submit bids that contemplate a single purchase price for the relevant hospital’s or hospitals’ operations and real estate combined.”
The complaint, which accused MPT of interfering in the bidding and sales process, seeks a judgment “allocating the amount of value attributable to hospital operations and other estate assets, on one hand, and hospital real estate, on the other.”
A proposed agreement filed last week said Orlando Health would pay $439 million in cash for Melbourne Regional Medical Center, Rockledge Regional Medical Center and Sebastian River Medical Center, though the amount could be adjusted based on a series of factors.
Orlando Health is designated as what is known in bankruptcy cases as a “stalking horse bidder,” which sets an initial bid. Other potential buyers of the three hospitals face a Monday deadline for submitting bids, according to a document filed in U.S. Bankruptcy Court in the Southern District of Texas.
Dallas-based Steward Health Care filed for Chapter 11 bankruptcy in May. In addition to Florida, it has operated hospitals in Arizona, Arkansas, Louisiana, Massachusetts, Ohio, Pennsylvania and Texas.
Melbourne Regional Medical Center and Rockledge Regional Medical Center are in Brevard County, while Sebastian River Medical Center is in Indian River County. In addition to those hospitals, Steward owns Coral Gables Hospital, Hialeah Hospital, North Shore Medical Center and Palmetto General Hospital in Miami-Dade County and Florida Medical Center in Broward County.
Steward in 2021 acquired the Miami-Dade and Broward hospitals from Tenet Healthcare Corp.
How to Fix TypeError: ‘Int’ Object Is Not Callable in Python
TypeError: ‘int’ object is not callable occurs in Python when an integer is called as if it were a function. Here’s how to fix it.

The Python “TypeError: ‘int’ object is not callable” error occurs when you try to call an integer (int object) as if it was a function. Overriding functions , and calling them later on, is the most common cause for this TypeError.
TypeError: ‘Int’ Object Is Not Callable Solved
The most common cause for TypeError: ‘int’ object is not callable is when you declare a variable with a name that matches the name of a function. Such as defining a variable named sum and calling sum() :
The best solution is to rename the variable, in this case sum , as follows:
Here’s what the error message looks like:
In the simplest terms, this is what happens:
Additionally, if you accidentally put an extra parenthesis after a function that returns an integer, you’ll get the same TypeError :
In the above example, the round() function returns an int value, and having an extra pair of parenthesis means calling the return integer value like function.
What Causes the TypeError: ‘Int’ Object Is Not Callable?
There are four common scenarios that create the “‘int’ object is not callable” error in Python. This includes:
- Declaring variable with a name that’s also the name of a function.
- Calling a method that’s also the name of a property.
- Calling a method decorated with @property.
- Missing a mathematical operator.
More on Python Python List and List Manipulation Tutorial
How to Solve TypeError “‘Int’ Object Is Not Callable” in Python
Let’s explore how to solve each scenario with some examples.
1. Declaring a Variable With a Name That’s Also the Name of a Function
A Python function is an object like any other built-in object, such as int , float , dict and list , etc.
All built-in functions are defined in the builtins module and assigned a global name for easier access. For instance, a call to sum() invokes the __builtins__.sum() function internally.
That said, overriding a function, accidentally or on purpose, with another value is technically possible.
For instance, if you define a variable named sum and initialize it with an integer value, sum() will no longer be a function.
If you run the above code, Python will produce a TypeError because 0, the new value of sum, isn’t callable.
This is the first thing to check if you’re calling a function, and you get this error.
You have two ways to fix the issue:
- Rename the variable sum .
- Explicitly access the sum function from the builtins module ( __bultins__.sum ).
The second approach isn’t recommended unless you’re developing a module. For instance, if you want to implement an open() function that wraps the built-in open() :
In almost every other case, you should always avoid naming your variables as existing functions and methods. But if you’ve done so, renaming the variable would solve the issue.
So, the above example could be fixed like this:
Here’s another example with the built-in max() function:
To fix it, we rename the max variable name to max_value :
Long story short, you should never use a function name (built-in or user-defined) for your variables.
Now, let’s get to the less common mistakes that lead to this error.
2. Calling a Method That’s Also the Name of a Property
When you define a property in a class constructor, any further definitions of the same name, such as methods, will be ignored.
Since we have a property named code, the method code() is ignored. As a result, any reference to the code will return the property code. Obviously, calling code() is like calling 1() , which raises the TypeError.
To fix it, we need to change the method name:
3. Calling a Method Decorated With @property Decorator
The @property decorator turns a method into a “getter” for a read-only attribute of the same name.
You need to access the getter method without the parentheses:
More on Python Understanding Duck Typing in Python
4. Missing a Mathematical Operator
In algebra , we can remove the multiplication operator to avoid ambiguity in our expressions. For instance, a × b , can be ab, or a × (b + c) can become a(b + c) .
But not in Python!
In the above example, if you remove the multiplication operator in a × (b + c) , Python’s interpreter would consider it a function call. And since the value of a is numeric, an integer in this case, it’ll raise the TypeError.
So, if you have something like this in your code:
You’d have to change it like so:
Problem solved.
Frequently Asked Questions
What does the typeerror: ‘int’ object is not callable mean in python.
Python produces the TypeError: ‘int’ object is not callable when you try to call an integer (int object) as if it was a function. This most often occurs when you override a function and then attempt to call it later on. The error looks like this:
How do you fix the TypeError: ‘int’ object is not callable in Python?
The most common cause for TypeError: ‘int’ object is when a variable is declared with a name that matches the name of a function. There are two ways to fix it:
Recent Python Algorithms Articles
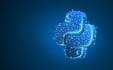
- Skip to main content
- Skip to search
- Skip to select language
- Sign up for free
- Português (do Brasil)
Object initializer
An object initializer is a comma-delimited list of zero or more pairs of property names and associated values of an object, enclosed in curly braces ( {} ). Objects can also be initialized using Object.create() or by invoking a constructor function with the new operator.
Description
An object initializer is an expression that describes the initialization of an Object . Objects consist of properties , which are used to describe an object. The values of object properties can either contain primitive data types or other objects.
Object literal syntax vs. JSON
The object literal syntax is not the same as the J ava S cript O bject N otation ( JSON ). Although they look similar, there are differences between them:
- JSON only permits property definition using the "property": value syntax. The property name must be double-quoted, and the definition cannot be a shorthand. Computed property names are not allowed either.
- JSON object property values can only be strings, numbers, true , false , null , arrays, or another JSON object. This means JSON cannot express methods or non-plain objects like Date or RegExp .
- In JSON, "__proto__" is a normal property key. In an object literal, it sets the object's prototype .
JSON is a strict subset of the object literal syntax, meaning that every valid JSON text can be parsed as an object literal, and would likely not cause syntax errors. The only exception is that the object literal syntax prohibits duplicate __proto__ keys, which does not apply to JSON.parse() . The latter treats __proto__ like a normal property and takes the last occurrence as the property's value. The only time when the object value they represent (a.k.a. their semantic) differ is also when the source contains the __proto__ key — for object literals, it sets the object's prototype; for JSON, it's a normal property.
Creating objects
An empty object with no properties can be created like this:
However, the advantage of the literal or initializer notation is, that you are able to quickly create objects with properties inside the curly braces. You notate a list of key: value pairs delimited by commas.
The following code creates an object with three properties and the keys are "foo" , "age" and "baz" . The values of these keys are a string "bar" , the number 42 , and another object.
Accessing properties
Once you have created an object, you might want to read or change them. Object properties can be accessed by using the dot notation or the bracket notation. (See property accessors for detailed information.)
Property definitions
We have already learned how to notate properties using the initializer syntax. Oftentimes, there are variables in your code that you would like to put into an object. You will see code like this:
There is a shorter notation available to achieve the same:
Duplicate property names
When using the same name for your properties, the second property will overwrite the first.
After ES2015, duplicate property names are allowed everywhere, including strict mode . You can also have duplicate property names in classes . The only exception is private properties , which must be unique in the class body.
- Method definitions
A property of an object can also refer to a function or a getter or setter method.
A shorthand notation is available, so that the keyword function is no longer necessary.
There is also a way to concisely define generator methods.
Which is equivalent to this ES5-like notation (but note that ECMAScript 5 has no generators):
For more information and examples about methods, see method definitions .
Computed property names
The object initializer syntax also supports computed property names. That allows you to put an expression in square brackets [] , that will be computed and used as the property name. This is reminiscent of the bracket notation of the property accessor syntax, which you may have used to read and set properties already.
Now you can use a similar syntax in object literals, too:
Spread properties
Object literals support the spread syntax . It copies own enumerable properties from a provided object onto a new object.
Shallow-cloning (excluding prototype ) or merging objects is now possible using a shorter syntax than Object.assign() .
Warning: Note that Object.assign() triggers setters , whereas the spread syntax doesn't!
Prototype setter
A property definition of the form __proto__: value or "__proto__": value does not create a property with the name __proto__ . Instead, if the provided value is an object or null , it points the [[Prototype]] of the created object to that value. (If the value is not an object or null , the object is not changed.)
Note that the __proto__ key is standardized syntax, in contrast to the non-standard and non-performant Object.prototype.__proto__ accessors. It sets the [[Prototype]] during object creation, similar to Object.create — instead of mutating the prototype chain.
Only a single prototype setter is permitted in an object literal. Multiple prototype setters are a syntax error.
Property definitions that do not use "colon" notation are not prototype setters. They are property definitions that behave identically to similar definitions using any other name.
Specifications
Specification |
---|
Browser compatibility
BCD tables only load in the browser with JavaScript enabled. Enable JavaScript to view data.
- Property accessors
- Lexical grammar
- Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers
- Advertising & Talent Reach devs & technologists worldwide about your product, service or employer brand
- OverflowAI GenAI features for Teams
- OverflowAPI Train & fine-tune LLMs
- Labs The future of collective knowledge sharing
- About the company Visit the blog
Collectives™ on Stack Overflow
Find centralized, trusted content and collaborate around the technologies you use most.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Get early access and see previews of new features.
use shorthand for object property assigment
I'm using the following code
Before I was using the or (||) I've used something like
this.props = Object.assign(this.props, props);
I've many fields which I need to assign is there a way to make it shorter?

- How about this: this.props = {...this.props, ...this.options} ? – Rajneesh Commented Jun 21, 2020 at 6:43
- 1 Why you give up the Object.assign way? – Oboo Cheng Commented Jun 21, 2020 at 7:12
- Does this answer your question? How can I merge properties of two JavaScript objects dynamically? – user120242 Commented Jun 21, 2020 at 8:33
- react shorthand for passing props – user120242 Commented Jun 21, 2020 at 8:33
- seems like a potential bug because values of 0 , "" and false will be replaced too – Slai Commented Jun 21, 2020 at 9:43
4 Answers 4
You can make use of spread operator here to unpack the values from other object, while keeping the first one as default.
var props={appName:'name', host:'host', appPath:'path'}; var options={appName:'UpdatedName', appPath:'pathUpdated'}; props = {...props, ...options}; console.log(props);
In case of undefine, I think you can iterate object using for..in loop, to settle the data:
var props={appName:'name', host:'host', appPath:'path'}; var options={appName:'UpdatedName', host:undefined, appPath:'pathUpdated'}; for(let item in options){ if(options[item]) props[item] = options[item]; } console.log(props);
- if options holds a falsy (e.g undefined) property, the || operator would have kept the props property while here you are setting that falsy property to props – grodzi Commented Jun 21, 2020 at 8:32
- but what happen if options is undfiend so that loop will not start – Beno Odr Commented Jun 21, 2020 at 13:00
Try the below
var props={appName:'name', host:'host', appPath:'path'}; var options={appName:'UpdatedName', appPath:'pathUpdated'}; props = Object.keys(props).reduce((target, key) => { target[key] = options[key] || props[key]; return target; }, {}); console.log(props);

- thanks, im using it in typescript and got error on the target[key] , TS7053: Element implicitly has an 'any' type because expression of type 'string' can't be used to index type '{}'. No index signature with a parameter of type 'string' was found on type '{}'. any idea how to resolve it? – Beno Odr Commented Jun 21, 2020 at 12:22
- I tried the same on typescript, working fine. Any particular framework? – Girish Sasidharan Commented Jun 21, 2020 at 13:55
- no, just using eslint recomended which is integreated with webstorm – Beno Odr Commented Jun 21, 2020 at 14:05
- tried the same on Angular 6 -> stackblitz.com/edit/copytaskshorthand , Typescript -> jsfiddle.net/boilerplate/typescript – Girish Sasidharan Commented Jun 21, 2020 at 14:11
You can do destruction:
As per your given snippet you just need to change the option in Object.assign().
This should be work for you.
Your Answer
Reminder: Answers generated by artificial intelligence tools are not allowed on Stack Overflow. Learn more
Sign up or log in
Post as a guest.
Required, but never shown
By clicking “Post Your Answer”, you agree to our terms of service and acknowledge you have read our privacy policy .
Not the answer you're looking for? Browse other questions tagged javascript node.js typescript or ask your own question .
- The Overflow Blog
- From PHP to JavaScript to Kubernetes: how one backend engineer evolved over time
- Featured on Meta
- We've made changes to our Terms of Service & Privacy Policy - July 2024
- Bringing clarity to status tag usage on meta sites
- What does a new user need in a homepage experience on Stack Overflow?
- Feedback requested: How do you use tag hover descriptions for curating and do...
Hot Network Questions
- Are Experimental Elixirs Magic Items?
- What sort of impact did the discovery that water could be broken down (via electrolysis) into gas have?
- TeXbook Exercise 21.10 Answer
- Is there anything that stops the majority shareholder(s) from destroying company value?
- Is there racial discrimination at Tbilisi airport?
- Why if gravity were higher, designing a fully reusable rocket would be impossible?
- When testing for normally distributed data, should I consider all variables before running shapiro.test?
- Do mini-humans need a "real" Saturn V to reach the moon?
- Existence of a mobius transformation
- Frequency resolution in STFT
- Can pedestrians and cyclists board shuttle trains in the Channel Tunnel?
- How to calculate APR and amount owed
- An integral using Mathematica or otherwise
- When a submarine blows its ballast and rises, where did the energy for the ascent come from?
- If the Collatz conjecture is undecidable, then it is true
- Will this be the first time, that there are more People an ISS than seats in docked Spacecraft?
- Mystery of 徐に: "slowly" or "suddenly"?
- Clarification on proof of the algebraic completeness of nimbers
- Is it possible to do physics without mathematics?
- How to extract code into library allowing changes without workflow overhead
- Can figere come with a dative?
- How can I get accessed to the data stored by gnome-pomodoro app?
- How do logic gates handle if-else statements?
- When was this photo taken?
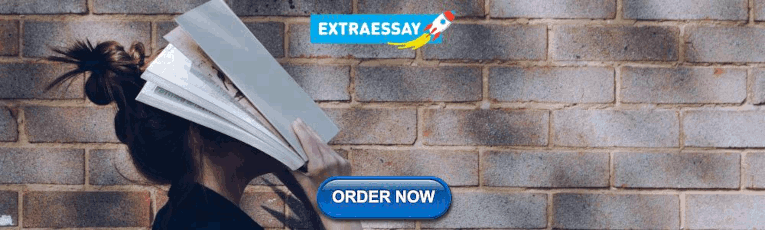
IMAGES
COMMENTS
Here's what I came up with: var obj = (obj = {}, obj[field] = 123, obj) It looks a little bit complex at first, but it's really simple. We use the Comma Operator to run three commands in a row: obj = {}: creates a new object and assigns it to the variable obj. obj[field] = 123: adds a computed property name to obj.
Defining methods. A method is a function associated with an object, or, put differently, a method is a property of an object that is a function. Methods are defined the way normal functions are defined, except that they have to be assigned as the property of an object. See also method definitions for more details.
In Alphabet.__init__, you don't have the effect of the setter but in Celsius.__init__ you have.. In other words in Celsius.__init__, you're setting the value of your private variable(you called it, they are not private by that meaning in other languages) through the setter, but in Alphabet.__init__ you're setting it directly.. So if you have any validation in your setter, it's not gonna work ...
Solution 1: Explicitly type the object at declaration time. This is the easiest solution to reason through. At the time you declare the object, go ahead and type it, and assign all the relevant values: type Org = {. name: string. } const organization: Org = {. name: "Logrocket" } See this in the TypeScript Playground.
The Object.assign() method only copies enumerable and own properties from a source object to a target object. It uses [[Get]] on the source and [[Set]] on the target, so it will invoke getters and setters. Therefore it assigns properties, versus copying or defining new properties.
JavaScript Object.defineProperty () The Object.defineProperty() method can be used to: Adding a new property to an object. Changing property values. Changing property metadata. Changing object getters and setters. Syntax:
Here, for example, const { p: foo } = o takes from the object o the property named p and assigns it to a local variable named foo. Assigning to new variable names and providing default values. A property can be both. Unpacked from an object and assigned to a variable with a different name. Assigned a default value in case the unpacked value is ...
11.1.1 Assignment #. We use the assignment operator = to assign a value value to a property .prop of an object obj: obj.prop = value. This operator works differently depending on what .prop looks like: Changing properties: If there is an own data property .prop, assignment changes its value to value. Invoking setters: If there is an own or ...
Object.defineProperty() allows developers to add or modify properties on objects. Unlike standard property assignment, this method enables the customization of property attributes. Syntax
The Object.assign() method copies properties from one or more source objects to a target object. Object.assign () copies properties from a source object to a target object. Object.create () creates an object from an existing object. Object.fromEntries () creates an object from a list of keys/values.
Object manipulation is one of the key concepts used by almost every JavaScript developer. While basic techniques like property assignment and object creation are essential, JavaScript offers more…
Object.defineProperty(obj, propName, propDesc) The primary purpose of this function is to add an own (direct) property to obj, whose attributes (writable etc., see below) are as specified by propDesc. The secondary purpose is to change the attributes of a property, including its value. Assignment. To assign to a property, one uses an expression ...
object[propertyName] = value; This does the exact same thing as the previous example. js. document["createElement"]("pre"); A space before bracket notation is allowed. js. document ["createElement"]("pre"); Passing expressions that evaluate to property name will do the same thing as directly passing the property name.
Primitives vs. Objects. As a review, let's recall the different primitive types and objects in JavaScript. Primitive types: Boolean, Null, Undefined, Number, BigInt (you probably won't see this much), String, Symbol (you probably won't see this much) Object types: Object, Array, Date, Many others.
In this video we take a look at Object Property Assignment in JavaScript, Codecademy's Learn JavaScript Assign Objects Properties. This is from Codecademy's...
If an object or an array contains other nested objects and arrays, we can use more complex left-side patterns to extract deeper portions. In the code below options has another object in the property size and an array in the property items. The pattern on the left side of the assignment has the same structure to extract values from them:
Orlando Health's proposed $439 million deal to buy three hospitals in Brevard and Indian River counties from Steward Health Care hits a snag.
So the global object will ultimately be searched for unqualified identifiers. You don't have to type globalThis.String; you can just type the unqualified String.To make this feature more conceptually consistent, assignment to unqualified identifiers will assume you want to create a property with that name on the global object (with globalThis. omitted), if there is no variable of the same name ...
TypeError: 'int' object is not callable occurs in Python when an integer is called as if it were a function. Here's how to fix it. ... The @property decorator turns a method into a "getter" for a read-only attribute of the same name. class Book: def __init__(self, book_code, book_title): self._title = book_title self._code = book_code ...
Object initializer. An object initializer is a comma-delimited list of zero or more pairs of property names and associated values of an object, enclosed in curly braces ( {} ). Objects can also be initialized using Object.create() or by invoking a constructor function with the new operator.
Assignment only creates another reference to the exact same object in memory. If you assign, then change by referencing one of the variable names, the other one will have changed too, since they're the same thing. Object.assign will assign all enumerable own properties of the second (and further) parameters to the first parameter, and will ...
Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers; Advertising & Talent Reach devs & technologists worldwide about your product, service or employer brand; OverflowAI GenAI features for Teams; OverflowAPI Train & fine-tune LLMs; Labs The future of collective knowledge sharing; About the company Visit the blog