TypeScript ESLint: Unsafe assignment of an any value [Fix]
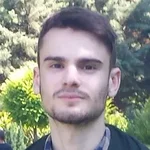
Last updated: Feb 29, 2024 Reading time Ā· 5 min
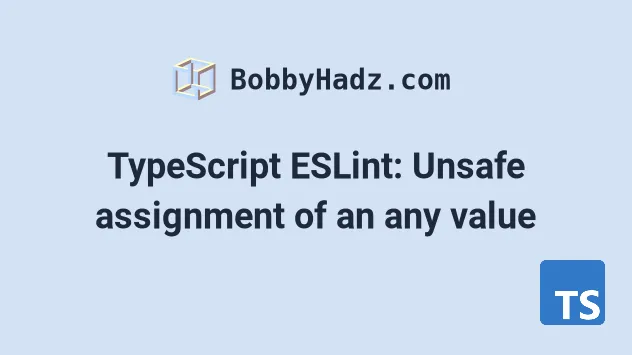
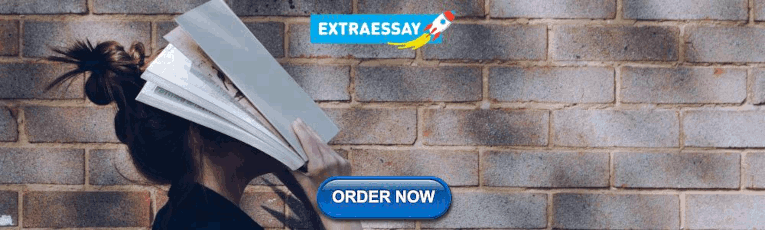
# TypeScript ESLint: Unsafe assignment of an any value
The error "@typescript-eslint/no-unsafe-assignment: Unsafe assignment of an any value." occurs when you assign a value with a type of any to a variable or a property.
To solve the error, set the variable to a specific type or disable the ESLint rule.
Here are some examples of when the ESLint error is raised.
All of the assignments above cause the error because the ESLint rule prevents you from assigning a value with an any type to a variable.
The any type effectively turns off type checking and should be used sparingly.
This article addresses 2 similar ESLint errors:
- @typescript-eslint/no-unsafe-assignment: Unsafe assignment of an any value.
- Unexpected any. Specify a different type. eslint@typescript-eslint/no-explicit-any
# Disabling the @typescript-eslint/no-unsafe-assignment ESLint rule
One way to get around the ESLint error is to disable the rule.
For example, the following comment disables the rule for 1 line.
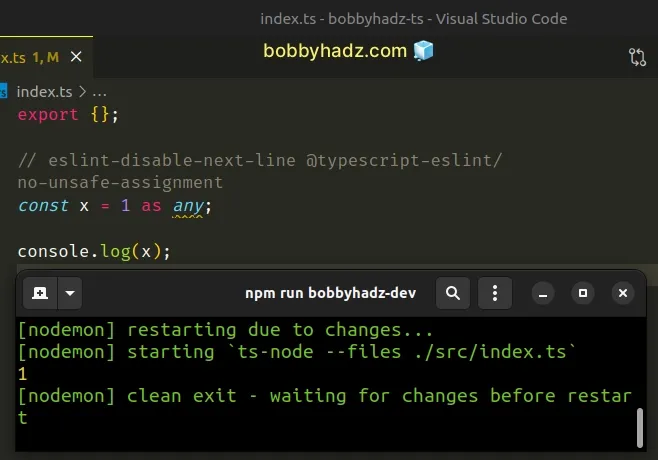
If you need to disable the @typescript-eslint/no-explicit-any rule for a single line, use the following comment.
If you need to disable multiple rules for a line, separate them by a comma.
If you need to disable the rule for the entire file, use the following comment.
If you need to disable the @typescript-eslint/no-explicit-any rule for the entire file, use the following comment instead.
You can disable both rules for the entire file by using the following comment.
If you want to disable the rules globally, add the following 2 rules to your .eslintrc.js file.
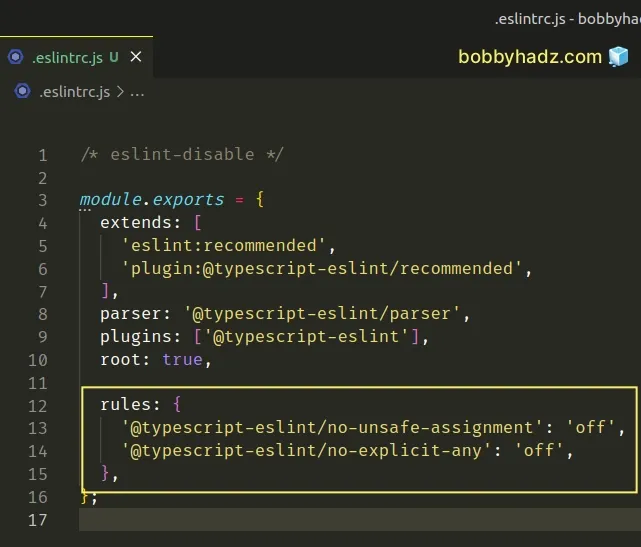
If you use a .eslintrc.json file, make sure to double-quote the keys and values.
# Setting the variable or property to unknown instead of any
Alternatively, you can set the variable or property to unknown to resolve the ESLint error.
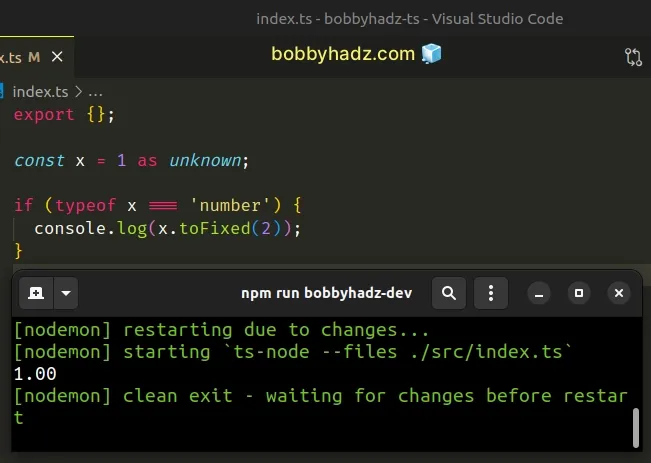
The unknown type is the type-safe counterpart of any .
When working with the unknown type, we basically tell TypeScript that we're going to get this value, but we don't know its type.
We are going to check with a couple of if statements to track the type down and use it safely.
I have written a detailed guide on how to check the type of a variable in TypeScript .
When using the unknown type, you have to use an if statement as a type guard to check the type of the variable before you are able to use any type-specific methods (e.g. string, array, object, etc).
# The error commonly occurs when parsing a JSON string
The error commonly occurs when parsing a JSON string with the JSON.parse() method.
The result variable stores a value of any type because TypeScript doesn't know the type of the value that is being parsed.
One way to resolve the issue is to use a type predicate .
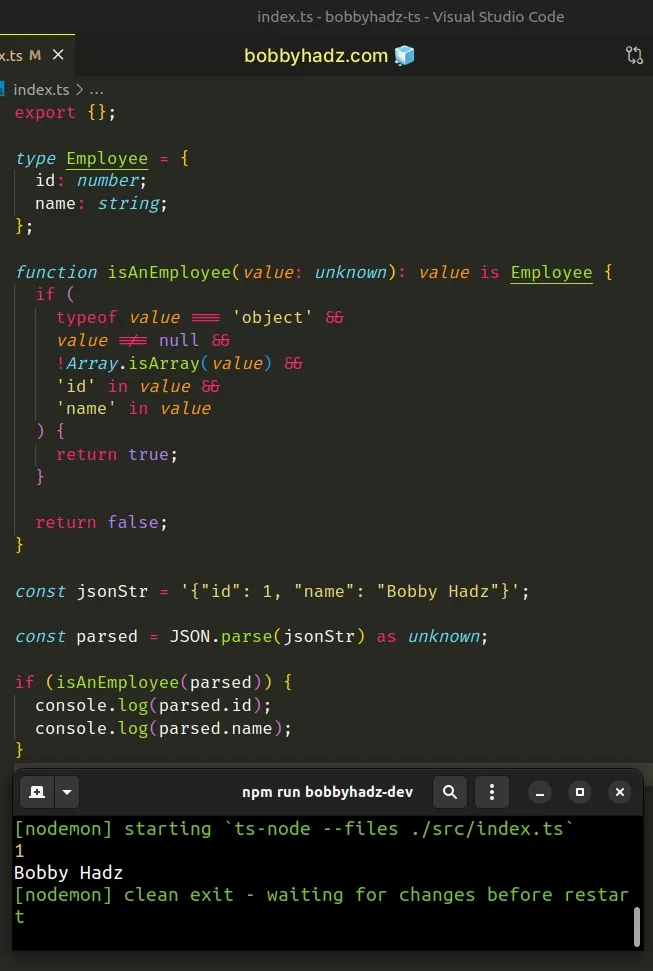
The value is Employee syntax is called a type predicate.
Our function basically checks if the passed-in value is compatible with an object of type Employee .
Notice that in the if block in which we called the isAnEmployee() function, the parsed variable is typed as Employee and we can access the id and name properties without getting TypeScript or ESLint errors.
I've written a detailed guide on how to check if a value is an object .
# Resolve the issue by typing the variable explicitly
You can also resolve the issue by typing the variable explicitly and removing the any type.
Here is an example of typing an object.
And here is an example of typing an array of objects.
You might have to use a type assertion, e.g. when parsing a JSON string.
In some rare cases, you might have to widen the type to unknown before using a type assertion to set a more specific type.
I've written detailed guides on:
- How to initialize a typed Empty Object in TypeScript
- Declare an Empty Array for a typed Variable in TypeScript
- How to add Elements to an Array in TypeScript
- Check if an Array contains a Value in TypeScript
- Check if a Value is an Array (of type) in TypeScript
- How to declare an Array of Objects in TypeScript
- How to declare a Two-dimensional Array in TypeScript
- Declare Array of Numbers, Strings or Booleans in TypeScript
- Create an Object based on an Interface in TypeScript
- Create a Type from an object's Keys or Values in TypeScript
- ESLint: Expected property shorthand object-shorthand [Fixed]
- 'X' should be listed in the project's dependencies, not devDependencies
- ESLint: Unexpected lexical declaration in case block [Fixed]
- ESLint couldn't find the config 'prettier' to extend from
- Import in body of module reorder to top eslint import/first
- ESLint: A form label must be associated with a control
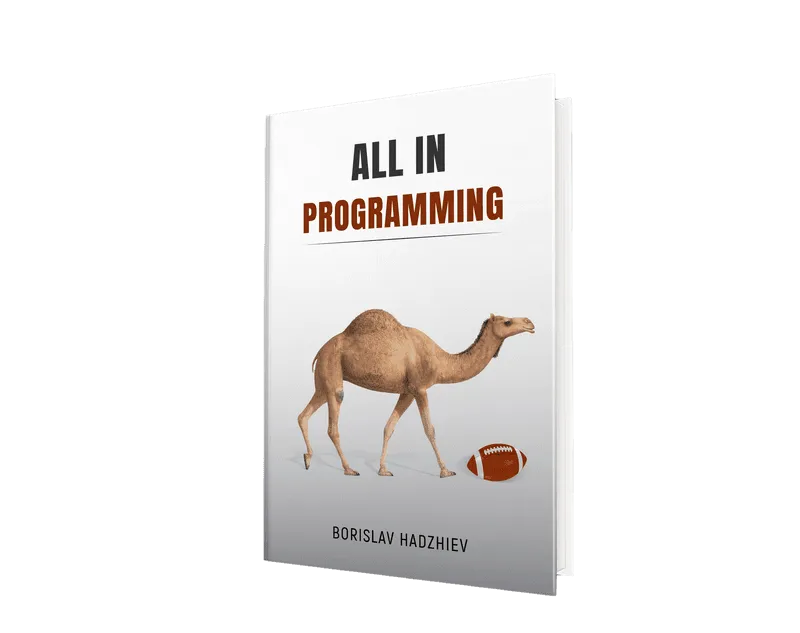
Borislav Hadzhiev
Web Developer
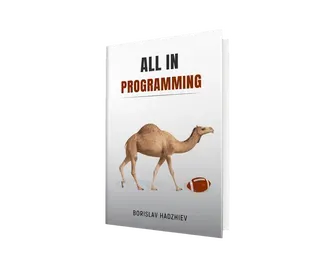
Copyright Ā© 2024 Borislav Hadzhiev
object-shorthand
The --fix option on the command line can automatically fix some of the problems reported by this rule.
Requires or disallows method and property shorthand syntax for object literals.
ECMAScript 6 provides a concise form for defining object literal methods and properties. This syntax can make defining complex object literals much cleaner.
Here are a few common examples using the ES5 syntax:
Now here are ES6 equivalents:
Rule Details
This rule enforces the use of the shorthand syntax. This applies to all methods (including generators) defined in object literals and any properties defined where the key name matches name of the assigned variable.
Each of the following properties would warn:
In that case the expected syntax would have been:
This rule does not flag arrow functions inside of object literals. The following will not warn:
The rule takes an option which specifies when it should be applied. It can be set to one of the following values:
- "always" (default) expects that the shorthand will be used whenever possible.
- "methods" ensures the method shorthand is used (also applies to generators).
- "properties" ensures the property shorthand is used (where the key and variable name match).
- "never" ensures that no property or method shorthand is used in any object literal.
- "consistent" ensures that either all shorthand or all long-form will be used in an object literal.
- "consistent-as-needed" ensures that either all shorthand or all long-form will be used in an object literal, but ensures all shorthand whenever possible.
You can set the option in configuration like this:
Additionally, the rule takes an optional object configuration:
- "avoidQuotes": true indicates that long-form syntax is preferred whenever the object key is a string literal (default: false ). Note that this option can only be enabled when the string option is set to "always" , "methods" , or "properties" .
- "ignoreConstructors": true can be used to prevent the rule from reporting errors for constructor functions. (By default, the rule treats constructors the same way as other functions.) Note that this option can only be enabled when the string option is set to "always" or "methods" .
- "avoidExplicitReturnArrows": true indicates that methods are preferred over explicit-return arrow functions for function properties. (By default, the rule allows either of these.) Note that this option can only be enabled when the string option is set to "always" or "methods" .
avoidQuotes
Example of incorrect code for this rule with the "always", { "avoidQuotes": true } option:
Example of correct code for this rule with the "always", { "avoidQuotes": true } option:
ignoreConstructors
Example of correct code for this rule with the "always", { "ignoreConstructors": true } option:
avoidExplicitReturnArrows
Example of incorrect code for this rule with the "always", { "avoidExplicitReturnArrows": true } option:
Example of correct code for this rule with the "always", { "avoidExplicitReturnArrows": true } option:
Example of incorrect code for this rule with the "consistent" option:
Examples of correct code for this rule with the "consistent" option:
Example of incorrect code with the "consistent-as-needed" option, which is very similar to "consistent" :
When Not To Use It
Anyone not yet in an ES6 environment would not want to apply this rule. Others may find the terseness of the shorthand syntax harder to read and may not want to encourage it with this rule.
Related Rules
- no-useless-rename
Further Reading
- https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Operators/Object_initializer
This rule was introduced in ESLint 0.20.0.
- Rule source
- Test source
- Documentation source

DEV Community

Posted on Jan 19, 2023
Destructuring Assignment and Shorthand Properties/Methods - Javascript
Hey dude, how are you?!
Javascript has a lot of interesting things and I'd like to talk about two specifically!
Destructuring Assignment and Shorthand Properties/Methods , let's talk about it?!
š Destructuring Assignment
The Destructuring Assignment is a powerful syntax to unpack an array, object and create distinct variables with the value, take a look:
āµ Destructuring Assignment: Array
āµ destructuring assignment: object, š shorthand properties.
Shorthand Properties released in ES6 makes the construction of the object simpler because when we have it with a property and a value and both have the same value we can omit the "value" and just declare the property, see the example below:
āµ Old mode:
āµ new mode:, š shorthand method names.
With Shorthand Method Names we can omit the function word, for example:
That's all guys! it gets more and more beautiful that we take to learn and practice!
I hope you guys like it and enjoy the article!
If you have a question, suggestion or anything else, please leave a comment!
See you later guys!
Top comments (0)
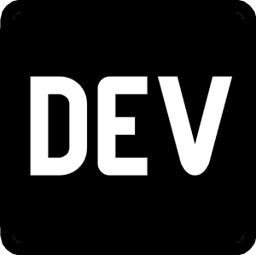
Templates let you quickly answer FAQs or store snippets for re-use.
Are you sure you want to hide this comment? It will become hidden in your post, but will still be visible via the comment's permalink .
Hide child comments as well
For further actions, you may consider blocking this person and/or reporting abuse

My Personal Experience Working in Big Tech - How to Avoid the Stress
Juan Emilio - Jul 28

Why Businesses Choose .NET Cross Platform Development?
Tarush Arora - Jul 28

My Journey to Becoming a Next.js Developer (and Beyond)
YGN - Aug 1
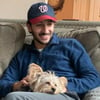
Retrieval Augmented Self-Reasoning Language Model Enhances Understanding
Mike Young - Aug 1
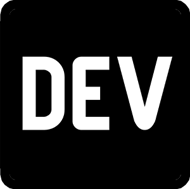
We're a place where coders share, stay up-to-date and grow their careers.
Shorthand Property and Method Names in JavaScript | ES6
ES6 introduced two new features to make objects more concise - Shorthand Properties and Shorthand Method Names.
Shorthand Properties
With Shorthand Properties, whenever you have a variable which is the same name as a property on an object, when constructing the object, you can omit the property name.
What that means is that code that used to look like this,
can now look like this.
Shorthand Method Names
Now, what if one of those properties was a function?
A function that is a property on an object is called a method. With ES6's Shorthand Method Names, you can omit the function keyword completely. What that means is that code that used to look like this,
can now look like this
Both Shorthand Properties and Shorthand Method Names are just syntactic sugar over the previous ways we used to add properties to an object. However, because they're such common tasks, even the smallest improvements eventually add up.
Before you leave
I know, another newsletter pitch - but hear me out. Most JavaScript newsletters are terrible. Whenās the last time you actually looked forward to getting one? Even worse, whenās the last time you actually read one? We wanted to change that.
We call it Bytes , but others call it their favorite newsletter .
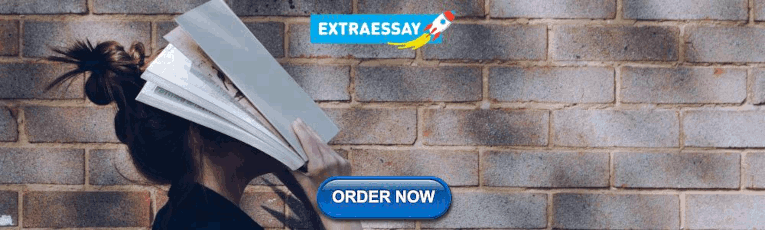
Delivered to 216,108 developers every Monday and Thursday
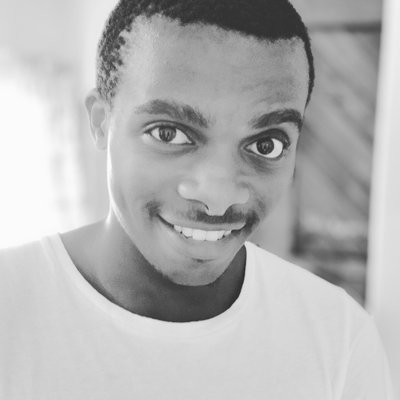
@ sduduzo_g
This is the first ever newsletter that I open a music playlist for and maximize my browser window just to read it in peace. Kudos to @uidotdev for great weekly content.
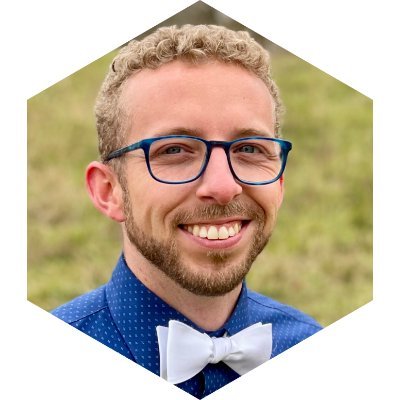
Brandon Bayer
The Bytes newsletter is a work of art! Itās the only dev newsletter Iām subscribed too. They somehow take semi boring stuff and infuse it with just the right amount of comedy to make you chuckle.
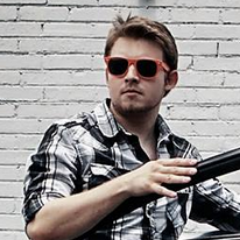
John Hawley
@ johnhawly
Bytes has been my favorite newsletter since its inception. Itās my favorite thing I look forward to on Mondays. Goes great with a hot cup of coffee!
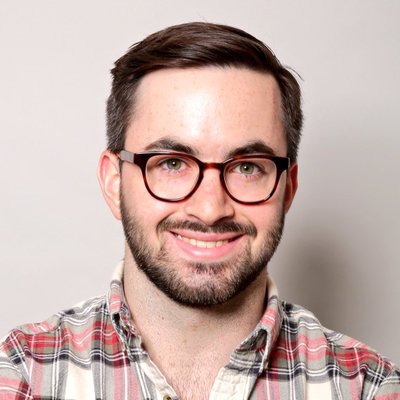
Garrett Green
@ garrettgreen
I subscribe to A LOT of dev (especially JS/TS/Node) newsletters and Bytes by @uidotdev is always such a welcomed, enjoyable change of pace to most (funny, lighthearted, etc) but still comprehensive/useful.
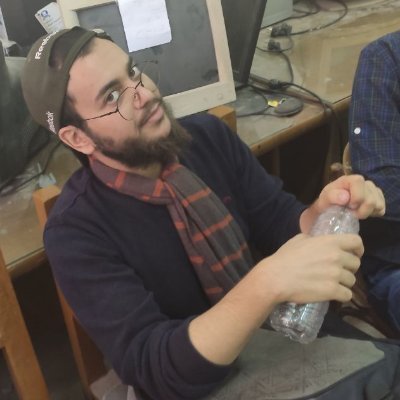
@ mhashim6_
Literally the only newsletter Iām waiting for every week.
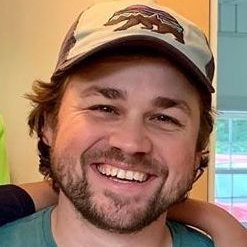
Grayson Hicks
@ graysonhicks
Bytes is the developer newsletter I most look forward to each week. Great balance of content and context! Thanks @uidotdev.
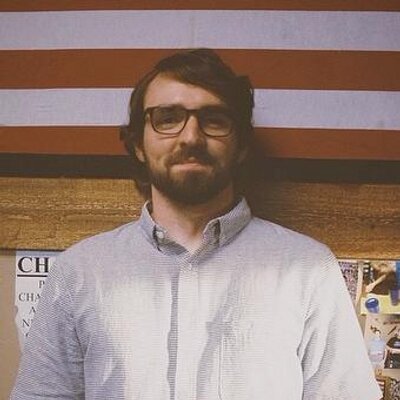
Mitchell Wright
@ mitchellbwright
I know Iāve said it before, but @tylermcginnis doesnāt miss with the Bytes email. If youāre a developer, you really need to subscribe
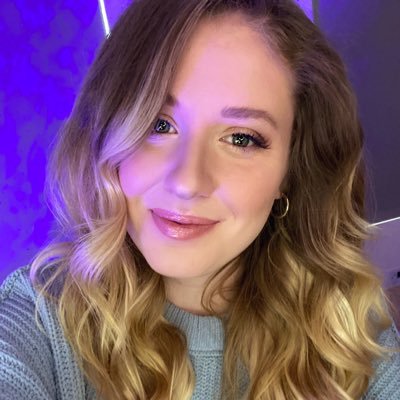
Ali Spittel
Can I just say that I giggle every time I get the @uidotdev email each week? You should definitely subscribe.
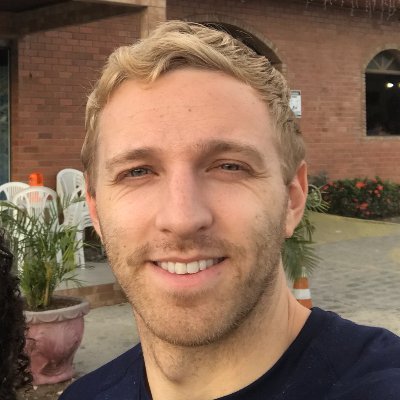
@ thefinnomenon
Every JavaScript programmer should be subscribed to the newsletter from @uidotdev. Not only do they manage to succinctly cover the hot news in the JavaScript world for the week but it they manage to add a refreshing humor to it all.

- Performance
- Announcements
- cfexchangemail
- cfhtmltopdf
- CFML Reference
- cfprocparam
- cfspreadsheet
- ColdFusion 10
- ColdFusion 11
- ColdFusion 2016
- ColdFusion 2018
- ColdFusion 20121
- ColdFusion Archives(or CAR)
- ColdFusion Mobile
- Enhancement
- Iconography
- Installation
- JDBC Connection
- JSON Backward Compatibility
- Language Enhancements
- Member Functions
- New feature
- New features
- QueryExecute
- serializeJSON
- session - less site
- SOLR Search
- Spreadsheet
- Usability Issues
- Application Datasource
- Application Monitoring
- Application Performance
- Distributed
- Performance Monitoring Toolset
- ColdFusion Summit Survey
- ColdFusion Webinar
- Developer week
- Govt Summit
- Adobe Developer Connection
- Amazon AWS Webinar
- Builder Plugin
- ColdFusion Community Portal
- ColdFusion seminar
- End of Sale
- Learn CF in a Week
- Linux Download
- Marketing Manager
- Migration Guide
- Online ColdFusion Meetup
- Windows 2016 Support
- Windows 2019 Support
- Adobe Certification
- Getting Started
- Discussions

This blog contains details about DeStructuring Assignment.
The Destructuring Assignment syntax is a way to assign the values from Arrays and Structs( Complex Objects ), into distinct variables.
Conventional way of doing it is to store the object and then retrieve it using the JSON Object API calls. However using DeStructuring , it becomes very simple, where just by writing a line of code, one can extract the relevant attributes and choose to ignore the other ones.
Note: The above defined syntax of [variable1, variable2, …………, variableN] =Ā [value1, value2, ……., valueN]Ā ,Ā is written for the sake of simplicity. This syntax can be as complex as possible.
For example here theĀ LVAL Ā i.eĀ [variable1, variable2, …………, variableN] , can be substituted with complexĀ values like Array/Struct elements or even a complex Object.
Similarly theĀ RVALĀ i.eĀ [value1, value2, ……., valueN] , can be substituted by Array/Struct elements as well as Array/Struct References or even a complex Object.
Lets get into more details and try to simulate the different scenarios through examples and see how we can write some short, concise and more powerful syntax using this feature.
- Multi-Initializer Statements with same #LVAL and #RVAL
- Multi-InitializerĀ Statements with RVAL as an Array Reference
- Multi-Initializer Statements by ignoring extra RVALs
- Multi-Initializer Statements to ignore some values from RVAL
- Multi-Initializer Statements using Rest Operator(…)
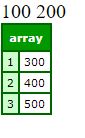
- Complex Multi-Initializer Statements
- Swapping Values
Object Destructuring :Ā Up till now, we have mostly seen the examples of ArrayInitializers as well as complex DeStructuring. DeStructuring is also supported for Structs as well as Complex Objects. Lets take some examples to simulate Object Destructuring and how we can leverage this functionality for Structs.
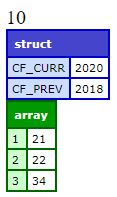
- Multi-Initializer Statements using Object Reference
- Multi-Initializer Statements with LVAL Object having default Values
- Nested Object Destructuring:Ā Using this one can initialize attributeValues from childObjects within ParentObject.

- Rest Operator Support in Objects Destructuring Multi-Initializer Statements
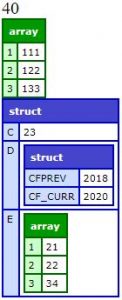
- Ignoring RVAL in Objects Destructuring
- Destructuring Support in local variables using var keyword
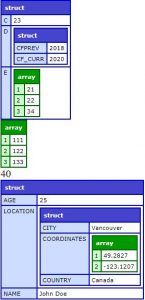
- Destructuring Support using Final keyword in Global as well as Local Scope

- Object Destructuring static keyword support :Ā Object Destructuring can also be leveraged in components using the static keyword. A sample code-snippet is as shown below.

This is a great feature, love using it in JavaScript, but the CF implementation misses it’s most powerful implementation and that is using it with function parameters. One should be able to call a function and pass a struct of named params with destructuring notation and have the inputs mapped. Example: var bar = {opt1: 1, opt2: 2, opt3:3}; function foo(required numeric opt1, any …opts){ writedump(arguments); }
foo({bar});
Expected output: { opt1: 1, opts: {opt2: 2, opt3: 3} }
I hope this makes sense. It is how I use this functionality in JS.

Thanks for sharing the observation. And while someone at Adobe MAY see this and respond, you would do better to file this also as a feature request at https://tracker.adobe.com .Ā Adobe really does pay attention to that (and sometimes to here).
Also, if you do that, you may want to help those not familiar with the implementation in JS (or the feature in general) by showing a working example in JS, such as could be could run at jsfiddle.net. That could help some better connect the dots of how what you propose does indeed work in JS.
Thanks Charlie, MDN already has examples of object destructuring in function parameters in their docs. https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Operators/Destructuring_assignment#object_destructuring
I thought youĀ areĀ someone at Adobe At least that’s the impression I have had from the last couple of developer conferences. I could be wrong. Anyway, I will submit it in the tracker.
Looks like someone made this suggestion in 2019 and they marked it as “fixed”.
https://tracker.adobe.com/#/view/CF-4205217
Nope, I donāt work for Adobe. I do work with them, as an ACP (Adobe Community Professional), and I may evangelize (and even do apologetics) on their behalf, but nope, not an employee. As always, Iām just trying to help.
BTW, whenever I speak, I clearly indicate that I am an independent consultant, on the front slide, then later when I introduce myself after the intro to the talk, and then also on the bottom of all my slides. Iād never intend (at all) to convey or leave open the impression that I work for Adobe (or anyone, but myself).
About that MSDN page, I wonder if to meant to share another.Ā That one does certainly discuss destructuring assignment, but I didn’t see any that showed its use in passing function arguments as you proposed. If there is another, that would be a help for many, I’m sure.
Look for ‘Unpacking fields from objects passed as a function parameter’ as a simple example.
Using spread in function calls: https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Operators/Spread_syntax#spread_in_function_calls
Interesting about the existing tracker ticket. And you added your comment asking about it being fixed there, and I offered a possible explanation.Ā But in case people here don’t go there, let me offer it (and a bit more) here:
The info from Adobe also indicated “For2022Triage”, which is certainly interesting to see. It suggests perhaps maybe the “fix” is coming in a CF2022. I agree they could be more clear, as that’s in the “component” field rather than the update/build. Perhaps they have to do this because there is not technically yet a cf2022.
And I’d heard some other rumblings that the next CF version would be called that (or would come out this year), so we shall see, on both fronts. š
Also, you offered there a link to a cffiddle example you created. I’m sharing that also for readers here, who may not have gone there. Also, about that example, it does not “fail”. You may want to revise it or clarify better what output yo unexpected it to produce.
Finally, of course, the folks there wouldn’t know about our discussions here, so I added a link there pointing to this. Such cross-posting of discussions can get messy, but I’m just trying to help given what you’ve offered.
Thanks for the additional feedback.
https://codepen.io/Anthony-Kolka/pen/WNZMZZg
Ah, ok (on that “unpacking” example). I was focusing more on the rest (“…”) aspect in your first example here and didn’t see that there.
But I see now how you’d hope to be able to name a struct key as the arg of a function, and pass the function the struct and have it figure it out/unpack it. I understand now, and hopefully this example (on top of yours and Abram’s in the tracker ticket) may help Adobe see what you want and deliver that in that seemingly upcoming enhancement.

So pretty interesting and I’ll admit that some of this went over my head and made me think hard about what was going on.Ā I’d be interested in seeing a real world example of how you would use this if you were able to put one together.Ā This is pretty abstract and I think it’d help me grasp it more firmly seeing it in a real world scenario.Ā That being said I think I can see how I may could use this so will start trying to play.
Also I am assuming this is CF2021 specific?Ā On CFFiddle the code would only run with 2021 selected so may be worthy of noting in the description unless I am incorrect that is.
Grae, yep, this is new to cf2021. And I can relate to your sentiment, having felt similarly when I first saw the feature and similar examples in the pre-release.
Fwiw, I will say that the feature is a generic one, and if you Google on it youāll find itās supported by other languages like Javascript, Python, etc.
And if you find resources on using the feature with those, the examples are similar, showing āhow it worksā but not so much āwhy would one botherā. I suspect they figure people can be left to connect the dots for the various ways one might leverage the capabilities.
But fwiw, as I kept looking for anything more, I did find this which you may appreciate :
https://www.smashingmagazine.com/2019/09/reintroduction-destructuring-assignment/
No, itās not cf-specific, but again neither is the feature. Hope it may help.
For real world examples check out React’s state implementation. Especially Redux.
You must be logged in to post a comment.

No credit card required.

Why am I Getting an Error After Copying a Working Step: "Shorthand Property Assignments are Valid Only in Destructuring Patterns (22:22)"?
This topic was automatically generated from Slack. You can find the original thread here .
hi. I had a working step that I copied and now I get error: Shorthand property assignments are valid only in destructuring patterns (22:22) DETAILS
Iām sorry to hear that youāre experiencing an issue with your code step. It seems like thereās a syntax error related to shorthand property assignments. To help you further, could you please share the relevant code snippet where the error is occurring? This will allow me to better understand the issue and provide a solution.
yes: import axios from āaxiosā;
export default defineComponent({ props: { Instance: { type: āstringā, label: āGreem-Api device Instance Numberā, default : ā1101795721ā, }, Green_token: { type: āstringā, label: āGreen-Api Device Tokenā, default : ā7bd245ff67294e609b7ff35bfe473f29ea49cc8a045b4c6fbdā, }, students_path: { type: āstringā, label: āStudents array step pathā, }, content: { type: āstringā, label: āWhatsapp Content Pathā, }, content_name: { type: āstringā, label: āWhatsapp Content Name Pathā, }, whatsapp: { type: āstringā, label: āWhatsapp Number Colum Nameā, }, base_uuid: { type: āstringā, label: āBase Uuidā, }, base_token: { type: āstringā, label: āBase Tokenā, }, logs_table_name: { type: āstringā, label: āLogs Table Nameā, default : ā××× ×©×××××Ŗā, }, message_type: { type: āstringā, label: ā×ש×× ××צ××× ××Ŗ ×”×× ×××××¢× ×ש××××ā, default : āSendMessageā, }, },
async run({ steps }) { const apiUrl = https://api.green-api.com/waInstance1101795721/SendMessage/7bd245ff67294e609b7ff35bfe473f29ea49cc8a045b4c6fbd/ ; const students = this .students_path; const seatableApiUrl = https://cloud.seatable.io/dtable-server/api/v1/dtables/8a3c2b05-24af-4125-ba10-93ad4a730b1f/rows/ ;
//const students = steps.get_activ_students.$return_value; // const apiUrl = ā https://api.green-api.com/waInstance1101796351/SendMessage/af6a35aea200435aa8c83d09acd9f5e117cf832eb15d4b2094/ ā;
I found the issue in your code. Itās related to the shorthand property assignment for the linkPreview property in the axios.post call. You should change it to a proper key-value pair. Hereās the corrected part of your code: ``` try { await axios.post (apiUrl, { chatId, message, linkPreview: true }); console.log( API call made successfully. ); } catch (error) { console.error( Error making API call: , error); }
thanks, now itās working!
thanks, now itās working. Now I have other problem: the message that is sent via apicall in this step, has special characters like *, : etc. How can I avoid adding \ that is breaking links etc? Itās been added automaticly
Iām glad to hear that the previous issue is resolved! To address the issue with special characters and backslashes, you can use JavaScriptās encodeURIComponent() function to encode the message before sending it in the API call. Hereās the updated part of your code: ``` try { const encodedMessage = encodeURIComponent(message); await axios.post (apiUrl, { chatId, message: encodedMessage, linkPreview: true, }); console.log( API call made successfully. ); } catch (error) { console.error( Error making API call: , error); }
not good at all. Now all the message is sent encoded and is unreadable
Apologies for the confusion. Instead of encoding the entire message, letās try to escape only the special characters that are causing issues. You can use a function like this to escape special characters: function escapeSpecialChars(str) { return str.replace(/([*:\\])/g, '\\$1'); } const escapedMessage = escapeSpecialChars(message); Now, use escapedMessage in your API call: ``` try { await axios.post (apiUrl, { chatId, message: escapedMessage, linkPreview: true, }); console.log( API call made successfully. ); } catch (error) { console.error( Error making API call: , error); }
now itās readable but itās worst. all the special characters are replaced. for example the message had this link:
before your fix it was like this: https:< //clyp.it/fyeqgvmh?token=ff9d44100b96aef331568259a11b33ec >
and now itās:
https\:< //clyp.it/fyeqgvmh?token=ff9d44100b96aef331568259a11b33ec >
I want it working!
I apologize for the confusion. It seems that the escaping method I provided earlier was not suitable for your use case. Letās try a different approach to handle the special characters in the message without breaking the links. Instead of escaping special characters, we can use a regular expression to match URLs in the message and replace them with a URL-encoded version. Hereās the updated part of your code: ``` function encodeUrlsInMessage(message) { const urlRegex = /https?://[^\s]+/g; return message.replace(urlRegex, (url) => encodeURIComponent(url)); } const encodedMessage = encodeUrlsInMessage(message); try { await axios.post (apiUrl, { chatId, message: encodedMessage, linkPreview: true, }); console.log( API call made successfully. ); } catch (error) { console.error( Error making API call: , error); }
not working! still: https:< //clyp.it/fyeqgvmh?token=ff9d44100b96aef331568259a11b33ec >
I apologize for the inconvenience caused by the previous solutions. It seems that the issue is not with the message itself, but with the way the API is handling the message. If possible, please share more information about the API youāre using, such as the API documentation or any specific requirements it has for handling special characters in the message. This will help me provide a more accurate solution for your issue.
this is the sending page in the Green-Api API docs. I donāt see anything rellevant but maybe you find: SendMessage # The method is aimed for sending a text message to a personal or a group chat. The message will be added to the send queue. Linked device not required when sending. Messages will be kept for 24 hours in the queue until account will be authorized The rate at which messages are sent from the queue is managed by Message sending delay parameter.
Request # To send a text message, you have to execute a request at:
For idInstance and apiTokenInstance request parameters, refer to Before you start section.

The maximum length of a text message is 4096 characters Request body example # Sending a message to a personal chat:
Sending a message to a group chat:
Sending a quoted message:
Response # Response parameters # ParameterTypeDescription idMessage string Sent message Id Response body example #
SendMessage errors # For a list of errors common to all methods, refer to Common errors section
Request examples # Python PHP cURL VBA Delphi
Previous Sending - overview
It looks like you want to send a message using the Green-API. To do this in a Pipedream workflow, you can create a code step with the following Node.js code: import { axios } from "@pipedream/platform"; export default defineComponent({ props: { idInstance: { type: "string", label: "Instance ID", }, apiTokenInstance: { type: "string", label: "API Token Instance", }, chatId: { type: "string", label: "Chat ID", }, message: { type: "string", label: "Message", }, }, async run({ $ }) { const url = `https://api.green-api.com/waInstance${this.idInstance}/sendMessage/${this.apiTokenInstance}`; const payload = { chatId: this.chatId, message: this.message, }; const headers = { "Content-Type": "application/json", }; const response = await axios($, { method: "POST", url, headers, data: payload, }); return response; }, }); Make sure to replace the idInstance , apiTokenInstance , chatId , and message props with the appropriate values. This code will send a message using the Green-API and return the response.
did you forgot?.. I already built this step, the problem is that links are sent broken. instead of Share, Embed & Upload Audio with Clyp
I get https:< //clyp.it/fyeqgvmh?token=ff9d44100b96aef331568259a11b33ec >
please reffer to all the chat above and give me new answers
I apologize for the confusion earlier. It seems that the issue is with the encoding of the URL. To fix this, you can try decoding the URL before sending it in the message. You can use the decodeURIComponent() function to decode the URL. Hereās an example of how to do this in your existing code: ``` const decodedUrl = decodeURIComponent(this.message); const payload = { chatId: this.chatId, message: decodedUrl, };
now it sent āundefindā. the content has a problem . can you fix this code: import axios from āaxiosā;
function encodeUrlsInMessage(message) { const urlRegex = /https?://[^\s]+/ g ; return message.replace(urlRegex, (url) => encodeURIComponent(url)); }
const decodedUrl = decodeURIComponent( this .message);
try { await axios.post (apiUrl, { chatId, message: decodedUrl, linkPreview: true , });
console.log( API call made successfully. ); } catch (error) { console.error( Error making API call: , error); }
AND in the inputs it looks that the link is still: https:< //clyp.it/fyeqgvmh?token=ff9d44100b96aef331568259a11b33ec >
I see that the issue might be related to the message variable. In your code, youāre using steps.get_hebrew_date_daily_contnet_working_dont_touch.$return_value[0]["×Ŗ××× ×××××”×פ"] as the message, but you also have a content prop defined. I assume you want to use the content prop instead. Hereās the updated code: import axios from "axios"; export default defineComponent({ // ...props definition... async run({ steps }) { const apiUrl = `https://api.green-api.com/waInstance${this.Instance}/SendMessage/${this.Green_token}/`; const students = this.students_path; const seatableApiUrl = `https://cloud.seatable.io/dtable-server/api/v1/dtables/${this.base_uuid}/rows/`; const chatId = `mailto:${this.whatsapp}@c.us`; const message = this.content; function encodeUrlsInMessage(message) { const urlRegex = /https?:\/\/[^\s]+/g; return message.replace(urlRegex, (url) => encodeURIComponent(url)); } const decodedUrl = decodeURIComponent(message); try { await [axios.post](http://axios.post)(apiUrl, { chatId, message: decodedUrl, linkPreview: true, }); console.log(`API call made successfully.`); } catch (error) { console.error(`Error making API call:`, error); } }, }); Please make sure to set the content prop value when running the component. If you still face any issues, let me know.
Now Green-Api responded error 466: ERR_BAD_REQUEST
- Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers
- Advertising & Talent Reach devs & technologists worldwide about your product, service or employer brand
- OverflowAI GenAI features for Teams
- OverflowAPI Train & fine-tune LLMs
- Labs The future of collective knowledge sharing
- About the company Visit the blog
Collectives⢠on Stack Overflow
Find centralized, trusted content and collaborate around the technologies you use most.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Get early access and see previews of new features.
Object property assignment with destructuring?
I'd like to use ES6 destructuring to assign properties of an object, but can't figure out the syntax.
>= ES6 (my own made-up, not-working syntax):
Is it possible to use destructuring assignment onto an object? What's the correct syntax?
EDIT: In my use case, dst is an object that existed well before needing to merge a subset of src 's properties; it is not a new Object created solely to 'borrow' from src .
- variable-assignment
- ecmascript-6
- This doesn't look much like destructuring to me, you could have just cloned the item. – MinusFour Commented Nov 16, 2015 at 19:28
- This is not a duplicate of stackoverflow.com/questions/25553910/… ; that question is not asking about assigning to one object a subset of properties from another, it's asking about creating a new object from a subset of properties from another. I'll edit my question to clarify that. – ericsoco Commented Nov 17, 2015 at 0:40
- @MinusFour I can't just clone or Object.assign() , because I don't want all of src 's properties. In my example, note I'm leaving behind c: 'baz' . – ericsoco Commented Nov 17, 2015 at 0:43
2 Answers 2
I think youāre going to have to repeat dst :

- 5 I tried this, but didn't have the parens around it. That caused a syntax error -- I now understand that was because the destructuring assignment was interpreted instead as an object literal. Thanks! – ericsoco Commented Nov 17, 2015 at 0:50
- I've tried this in node v5.6 with --harmony_shipping and it doesn't appear to work - "Invalid left-hand side in assignment" – Alnitak Commented Feb 23, 2016 at 11:59
- @Alnitak: V8 doesnāt support all of ES6. Works in Firefox. – Ry- ♦ Commented Feb 23, 2016 at 14:58
The cleanest approach IMO is as follows:
run the example in this codepen
Your Answer
Reminder: Answers generated by artificial intelligence tools are not allowed on Stack Overflow. Learn more
Sign up or log in
Post as a guest.
Required, but never shown
By clicking āPost Your Answerā, you agree to our terms of service and acknowledge you have read our privacy policy .
Not the answer you're looking for? Browse other questions tagged javascript variable-assignment ecmascript-6 or ask your own question .
- Featured on Meta
- We've made changes to our Terms of Service & Privacy Policy - July 2024
- Announcing a change to the data-dump process
Hot Network Questions
- What duration should I choose when applying for a 1 year multi entry Schengen visa?
- If an agreement puts some condition on a file, does the condition also apply to its content? (the content itself is under CC BY-SA)
- Crowdstrike IT outage flight cancellations and EU 261 compensation trend
- Boundedness of sum of sin(sin(n))
- pdflatex can't parse webman.tex
- Will this short-circuit protection circuit work?
- Setting output raster bit depth using more efficient method than copy raster
- Is believing that an object that you’re staring at won’t suddenly pop out of existence justifiable or unjustifiable?
- Can I replace the Sun with a non-nuclear equivalent?
- A problem in the application of numerical integration
- How to combine some ordered pairs
- Cubic Concatenation
- Can it be proven from pure logic that at least one thing exists?
- Count number display: "99+" vs full number
- On a stopover, a space tourist who is in fact a gamesharp joins what looks like a casino game
- Ratio of real world to risk-neutral density
- Terrain rising, controller not giving climb clearance... what to do?
- Running the plot of Final Fantasy X
- Why can't we prove SAT is NP complete just using the Tseytin Transformation?
- Can any analytic function be written as the difference of two monotonically increasing analytic functions?
- Is it possible for a flying superhuman to avoid detection in todays society?
- Is there a ring with zero divisors but no nilpotents?
- Lexicographically earliest permutation of the initial segment of nonnegative integers subject to divisibility constraints
- How to become Emperor of the Galaxy
Navigation Menu
Search code, repositories, users, issues, pull requests..., provide feedback.
We read every piece of feedback, and take your input very seriously.
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
- Notifications You must be signed in to change notification settings
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement . Weāll occasionally send you account related emails.
Already on GitHub? Sign in to your account
bug: Module parse failed: Shorthand property assignments are valid only in destructuring patterns #452
DiegoCantu commented Jun 23, 2023
./node_modules/next/dist/build/webpack/loaders/next-flight-client-module-loader.js |
The text was updated successfully, but these errors were encountered: |
tobySolutions commented Jun 24, 2023
Hey ! Can you remove the block and send a reproduction so we can check it out? |
Sorry, something went wrong.
DiegoCantu commented Jun 24, 2023
Sidebar.tsx.-.redy.-.Visual.Studio.Code.2023-06-24.10-42-25.mp4 |
aidenybai commented Jun 29, 2023
Hold on, does this work without block? ? Might be a conflict with react refresh |
tobySolutions commented Jun 29, 2023
? Might be a conflict with react refresh Hmm, the code looks kinda tricky to me too. |
DiegoCantu commented Jun 29, 2023 • edited Loading
This works perfectly fine without million block, actually this is existing code that I try to add million , also after million is stable for next.js, I'll try to add millions to 60+ react components, which...also already existing and work correctly, but most of my components have props similar to those shown in the error so I can't continue if at least this error is not solved |
DiegoCantu commented Jun 29, 2023
I created this repo to reproduce the issue: |
aidenybai commented Jul 1, 2023 • edited Loading
Seems it be an error for typescript parsing. If you remove BlockComponent = block<SidebarProps>(Sidebar);to: BlockComponent = block(Sidebar);
|
DiegoCantu commented Jul 1, 2023
is the same issue without props in block:
|
- š 1 reaction
tobySolutions commented Jul 1, 2023
Without props? Wait, why? Can you share your code? |
DiegoCantu commented Jul 2, 2023
without props to test that:
this is the repo: |
aidenybai commented Jul 3, 2023
Whoa, now that's weird. Let me get back to you soon |
tobySolutions commented Jul 6, 2023
Alright, thanks ! We'll get back to you soon for sure . |
aidenybai commented Jul 16, 2023
can you test with the latest release? |
DiegoCantu commented Jul 16, 2023
Now this is the new issue, and obviously Sidebar is defined: error src\app\components\Sidebar.tsx (57:23) @ _Sidebar (C:\Users\diego\Desktop\RWB\Million\million-test.next\server\webpack-runtime.js:33:42) |
github-actions bot commented Nov 3, 2023
This issue has been automatically marked as stale because it has not had recent activity. It will be closed if no further activity occurs within the next 7 days. |
No branches or pull requests
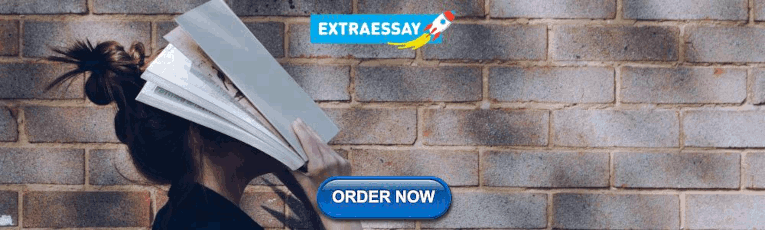
IMAGES
VIDEO
COMMENTS
An excerpt from eslint regarding the issue: Require Object Literal Shorthand Syntax (object-shorthand) - Rule Details. This rule enforces the use of the shorthand syntax. This applies to all methods (including generators) defined in object literals and any properties defined where the key name matches name of the assigned variable. Change
Note that this option can only be enabled when the string option is set to "always" or "methods". "methodsIgnorePattern" (string) for methods whose names match this regex pattern, the method shorthand will not be enforced. Note that this option can only be used when the string option is set to "always" or "methods".
For your 2nd issue, it's a typo, you're passing an object and y is a property, so it should be in:fly={{y: -200, .. No clue about your default export issue however, I've never had to explicitly export components.
Accessing an array element through a computed index will fall under the category object destructuring. The --fix option on the command line fixes only problems reported in variable declarations, and among them only those that fall under the category object destructuring. Furthermore, the name of the declared variable has to be the same as the ...
The foo property uses the longer syntax because there is no foo variable that is available in the scope.. All other object properties use the shorter syntax because variables with the same names are available. # Expected method shorthand. eslint object-shorthand You might also get the warning "Expected method shorthand. eslint object-shorthand" when you use the longer-form syntax to declare a ...
You signed in with another tab or window. Reload to refresh your session. You signed out in another tab or window. Reload to refresh your session. You switched accounts on another tab or window.
ESLint: Expected property shorthand object-shorthand [Fixed] 'X' should be listed in the project's dependencies, not devDependencies; ESLint: Unexpected lexical declaration in case block [Fixed] ESLint couldn't find the config 'prettier' to extend from; Import in body of module reorder to top eslint import/first
EDIT: forgot to mention, I looked at object-shorthand which only seems to be for literals, and prefer-destructuring which doesn't seem to have any options with respect to declaration shorthands. 1. Answered by mdjermanovic on Jul 6, 2022. const foo = {a: "foo"}
I read the doc, object-shorthand was a rule about object literals (object initializers). So I think making the rule covering destructuring assignments is an enhancement. Though I don't have any objection, I'd like to separate it to a new issue.
Requires or disallows method and property shorthand syntax for object literals. ECMAScript 6 provides a concise form for defining object literal methods and properties. This syntax can make defining complex object literals much cleaner. Here are a few common examples using the ES5 syntax: // properties var foo = {. x: x, y: y, z: z, };
š Shorthand Properties Shorthand Properties released in ES6 makes the construction of the object simpler because when we have it with a property and a value and both have the same value we can omit the "value" and just declare the property, see the example below: Old mode:
ES6 introduced two new features to make objects more concise - Shorthand Properties and Shorthand Method Names. With Shorthand Properties, whenever you have a variable which is the same name as a property on an object, when constructing the object, you can omit the property name. What that means is that code that used to look like this,
The destructuring syntax is used for assigning object and array entries to their own variables. For instance, we can write the following code to assign array entries to individual variables: const [a, b] = [1, 2]; Then, the destructuring assignment syntax assigns the entries to the variables located in the same position.
Please describe what the rule should do: Disallows references within object or array destructuring pattern values if they are not simple variables (if they are a property reference). Computed properties are still allowed as are default v...
Object Destructuring : Up till now, we have mostly seen the examples of ArrayInitializers as well as complex DeStructuring. DeStructuring is also supported for Structs as well as Complex Objects. Lets take some examples to simulate Object Destructuring and how we can leverage this functionality for Structs.
However in case of shorthand property assignments: ... Also note that the same problem exists with the destructuring pattern of for-of loop (perhaps, due to the same reasons): var s; for ({s = 5} of q) {} ... parse/check/emit shorthand property assignment in destructuring microsoft/TypeScript 5 participants Footer
You can find the original thread here. hi. I had a working step that I copied and now I get error: Shorthand property assignments are valid only in destructuring patte⦠This topic was automatically generated from Slack. You can find the original thread here. ... Shorthand property assignments are valid only in destructuring patterns (22:22 ...
Destructuring assignment to Object.property.property. Hot Network Questions How were East German citizens who defected to West Berlin able to travel to the rest of West Germany? (If at all) Does there exist a power series which sends every algebraic number in its radius of convergence to a rational number? ...
bug: Module parse failed: Shorthand property assignments are valid only in destructuring patterns #452. Closed DiegoCantu opened this issue Jun 23, 2023 Ā· 15 comments Closed bug: Module parse failed: Shorthand property assignments are valid only in destructuring patterns #452. DiegoCantu opened this issue Jun 23, 2023 Ā· 15 comments Assignees ...